请用C语言编写一个词法分析器代码
时间: 2024-09-27 13:08:10 浏览: 7
在C语言中编写词法分析器通常涉及到使用自定义数据结构和模式匹配来解析输入源代码,比如简单的标识符、关键字、运算符等。这通常是一个过程式的任务,并非完整的程序,因为词法分析器通常是作为其他工具(如编译器或解释器)的一部分来实现的。下面是一个非常基础的例子,展示如何使用C语言处理简单的英文单词:
```c
#include <stdio.h>
#include <ctype.h>
typedef enum {
TOKEN_ID,
TOKEN_NUMBER,
TOKEN_DELIMITER,
// 添加更多令牌类型...
} TokenType;
struct Token {
TokenType type;
union {
char* str; // 字符串类型的令牌
int num; // 数字类型的令牌
// 其他类型的令牌...
} data;
};
// 函数来读取并识别下一个令牌
Token next_token(char* input) {
Token token;
while (isspace(*input)) input++; // 跳过空格
if (*input == '#') { // 遇到注释,跳过至行尾
while (*input != '\n') input++;
return next_token(input);
}
if (isdigit(*input)) { // 处理数字
token.type = TOKEN_NUMBER;
do {
token.data.num = token.data.num * 10 + (*input - '0');
} while (isdigit(*(input++)));
if (*input == '.') { // 如果遇到小数点
token.data.num += *(input++) - '0';
while (isdigit(*(input++))) token.data.num *= 10;
}
} else if (isalpha(*input) || _isupper(*input)) { // 处理标识符
token.type = TOKEN_ID;
do {
token.data.str = realloc(token.data.str, strlen(token.data.str) + 2);
*(token.data.str++) = tolower(*input++);
} while (isalnum(*input));
*token.data.str = '\0'; // 结束字符串
} else if (*input == ',') { // 处理逗号
token.type = TOKEN_DELIMITER;
input++;
} else { // 处理其他未识别字符
printf("Unknown token: %c\n", *input);
token.type = TOKEN_ID; // 将其视为标识符
token.data.str = input;
input++;
}
return token;
}
int main() {
char input[] = "123, myVar # comment";
Token token;
while ((token = next_token(input)) != TOKEN_EOF) {
switch (token.type) {
case TOKEN_NUMBER:
printf("Number: %d\n", token.data.num);
break;
case TOKEN_ID:
printf("Identifier: %s\n", token.data.str);
break;
// ...添加对其他令牌类型的处理
}
}
return 0;
}
```
这个例子非常简单,实际的词法分析器会更复杂,能处理更多的语言特性。请注意,这只是一个起点,完整词法分析器需要考虑错误处理、结束标记(如`TOKEN_EOF`)、以及处理更复杂的语法元素。
相关推荐
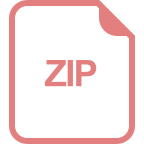
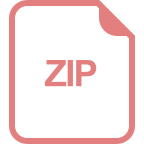
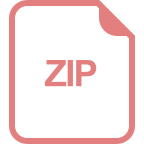














