编写c语言程序,输入一个字符串(可以包含数字,大小写字母,特殊符号等),统计该字符串中所有大写字母的个数
时间: 2024-10-24 22:13:56 浏览: 43
当你编写一个C语言程序来统计给定字符串中大写字母的数量时,你可以使用`ctype.h`库提供的函数来检查字符是否为大写字母。下面是一个简单的步骤和示例代码:
1. 包含头文件:
```c
#include <stdio.h>
#include <ctype.h>
```
2. 定义并初始化计数变量:
```c
int uppercase_count = 0;
```
3. 使用循环遍历字符串,并使用`isupper()`函数判断字符:
```c
char str[100]; // 假设字符串长度不超过100
printf("请输入一个字符串: ");
fgets(str, sizeof(str), stdin); // 从用户那里获取输入
for (int i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) {
uppercase_count++;
}
}
```
4. 输出结果:
```c
printf("大写字母的数量: %d\n", uppercase_count);
```
完整代码示例:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
int uppercase_count = 0;
char str[100];
printf("请输入一个字符串: ");
fgets(str, sizeof(str), stdin);
for (int i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) {
uppercase_count++;
}
}
printf("大写字母的数量: %d\n", uppercase_count);
return 0;
}
```
阅读全文
相关推荐
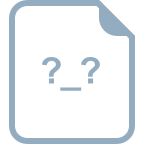
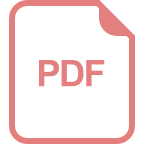



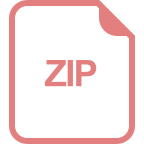
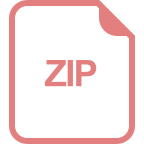
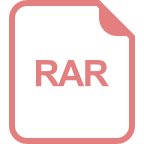
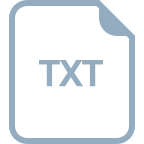
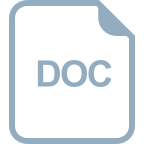
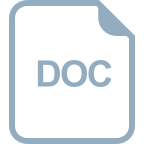





