光敏电阻单片机计数器
时间: 2024-12-26 13:19:36 浏览: 9
### 使用单片机和光敏电阻实现计数器电路设计及编程方法
#### 1. 硬件连接
为了构建基于单片机和光敏电阻的简单计数器,硬件部分主要由以下几个组件构成:
- STC15系列单片机作为核心控制单元;
- 光敏电阻用于检测环境光线强度变化;
- 数码管或其他显示设备来呈现当前计数值。
具体接线方式如下:
- 将光敏电阻一端接地(GND),另一端接到模拟输入引脚(例如P1口),并通过上拉电阻连接到电源VCC。
- 显示模块的数据线依次对应单片机I/O接口,以便于数据传输与刷新显示内容。
#### 2. 软件程序编写
针对上述提到的功能需求,在软件层面需完成以下几项工作:
##### 初始化设置
配置好定时器/计数器的工作模式以及中断优先级等参数;初始化ADC(模数转换)通道并开启相应的外部中断源。这部分可以通过调用特定库函数简化操作流程[^1]。
```c
#include <reg52.h>
sbit LED=P2^0;
void Timer_Init(void){
TMOD = 0x01;
TH0 = (65536-500)/256;
TL0 = (65536-500)%256;
ET0 = 1;
EA = 1;
TR0 = 1;
}
```
##### 主循环逻辑
不断读取来自光敏传感器经由ADC转化后的数字信号值,并依据预设阈值判断是否触发一次有效脉冲事件从而增加计数变量count。当达到一定条件时更新显示屏上的数据显示。
```c
unsigned int AD_Conversion(unsigned char ch) {
ADC_CONTR = 0x84 + ch; // Select channel and start conversion
while (!(ADSTAT & 0x01)); // Wait until conversion is complete
return ((unsigned int)(ADRESH << 8) | ADRESL);
}
int main(){
unsigned short adc_value;
unsigned long count = 0;
Timer_Init();
while(1){
adc_value = AD_Conversion(0); // Assuming the photoresistor connected to CH0
if (adc_value > LIGHT_THRESHOLD){ // Define a threshold value for detecting changes in light intensity.
++count;
// Process display update here...
delay_ms(DEBOUNCE_DELAY); // Debounce delay after each detection
}
}
}
```
##### 中断服务子程序(ISR)
每当发生溢出或捕捉到边沿跳变时进入该ISR处理过程,通常用来执行快速响应的任务比如重装载初值、累加次数统计量或是启动下一轮测量周期等活动。
```c
void External_Interrupt_Service_Routine() interrupt 0 using 1{
static bit flag = 0;
if(flag == 0){
flag = 1;
// Perform actions when rising edge detected, such as incrementing counter variable or toggling output pin state.
}else{
flag = 0;
// Handle falling edge event if necessary.
}
}
```
通过以上步骤即可搭建起一套完整的基于STC15单片机平台利用光敏元件特性来进行自动增减计数的应用实例.
阅读全文
相关推荐
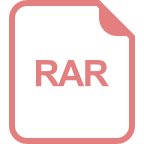
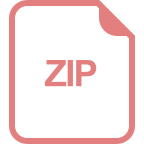
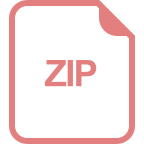
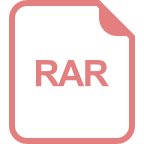
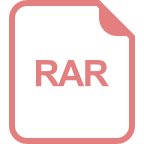
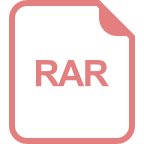
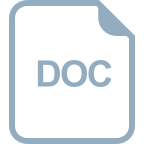
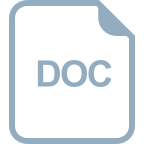
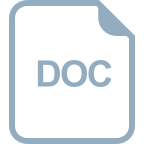
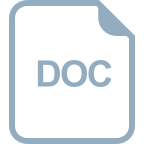

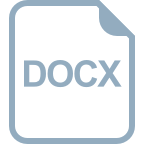
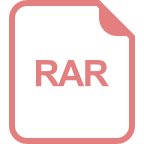
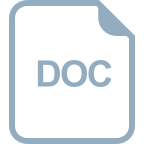
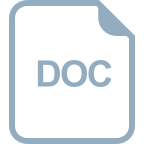
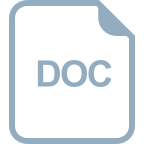
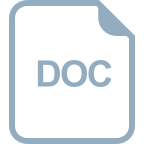
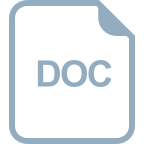

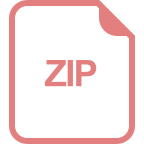