用DevCpp软件设计一个有序顺序表,要求:把有序顺序表L1[3,5,8,11]和L2[2,6,8,9,11,15,20]中的数据元素合并到L3,要求L3中的数据元素依旧保持有序。
时间: 2024-10-24 13:08:26 浏览: 20
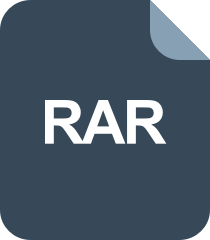
shunxubiao.rar_K3W_devc++shunxubiao_顺序表的基本操作
在Dev-C++中设计一个有序顺序表并合并两个列表,我们可以创建一个简单的结构体作为节点,然后定义一个函数来进行合并操作。这里是一个基本的步骤:
1. 首先,定义一个结构体Node来表示顺序表的节点:
```cpp
struct Node {
int data;
Node* next;
};
```
2. 定义一个顺序表类List,包含头指针head和一个合并函数mergeLists():
```cpp
class List {
private:
Node* head;
public:
// 构造函数、插入元素等其他成员函数...
void mergeLists(List& list2) {
if (list2.head == nullptr) return; // 如果list2为空,直接将list1复制过去
Node* current1 = head;
Node* current2 = list2.head;
while (current1 != nullptr && current2 != nullptr) {
if (current1->data <= current2->data) {
current1->next = current2;
current1 = current2;
current2 = current2->next;
} else {
current1->next = current2->next;
current1 = current2;
current2 = current2->next;
}
}
// 将list2剩余部分添加到list1的尾部
while (current2 != nullptr) {
current1->next = current2;
current1 = current1->next;
current2 = current2->next;
}
}
};
```
3. 使用这两个类,你可以创建和合并两个列表示例:
```cpp
int main() {
List L1({3, 5, 8, 11});
List L2({2, 6, 8, 9, 11, 15, 20});
// 合并L1和L2
L1.mergeLists(L2);
// 打印合并后的L3(注意,这里是打印节点的数据,而不是修改原列表)
Node* temp = L1.head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
return 0;
}
```
阅读全文
相关推荐
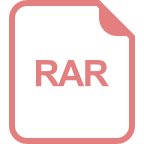





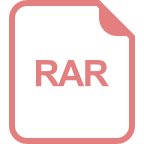


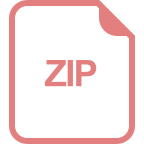
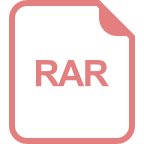
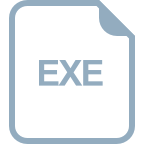
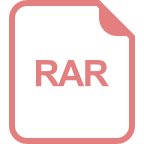
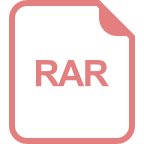
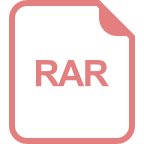
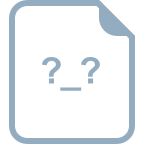