写一个Java程序实现摄氏温度和华氏温度转换
时间: 2024-10-29 20:23:59 浏览: 32
在Java中,我们可以创建一个简单的类来实现摄氏到华氏的温度转换以及华氏到摄氏的转换。这里是一个基础的示例:
```java
public class TemperatureConverter {
// 摄氏转华氏的公式
public static double celsiusToFahrenheit(double celsius) {
return (celsius * 9.0 / 5.0) + 32;
}
// 华氏转摄氏的公式
public static double fahrenheitToCelsius(double fahrenheit) {
return (fahrenheit - 32) * 5.0 / 9.0;
}
public static void main(String[] args) {
// 示例:输入摄氏温度并转换为华氏
double celsius = 25;
double fahrenheit = celsiusToFahrenheit(celsius);
System.out.println("Celsius: " + celsius + ", Fahrenheit: " + fahrenheit);
// 示例:输入华氏温度并转换为摄氏
double fahrenheitValue = 77; // 人体舒适的室温
double celsiusValue = fahrenheitToCelsius(fahrenheitValue);
System.out.println("Fahrenheit: " + fahrenheitValue + ", Celsius: " + celsiusValue);
// 动态调用转换方法,演示多态
String unit = "Celsius"; // 输入单位
double inputTemperature = 30;
if ("Celsius".equals(unit)) {
System.out.println("Converting from Celsius to Fahrenheit...");
double convertedTemp = celsiusToFahrenheit(inputTemperature);
System.out.println("Converted temperature is: " + convertedTemp + " F");
} else if ("Fahrenheit".equals(unit)) {
System.out.println("Converting from Fahrenheit to Celsius...");
double convertedTemp = fahrenheitToCelsius(inputTemperature);
System.out.println("Converted temperature is: " + convertedTemp + " C");
} else {
System.out.println("Invalid unit. Please enter 'Celsius' or 'Fahrenheit'.");
}
}
}
```
这个程序首先定义了两个静态方法,然后在`main`方法中展示了如何使用这两个方法进行温度转换,并展示了动态方法调用,即通过字符串来判断应该调用哪种类型的转换方法,体现了多态。
阅读全文
相关推荐
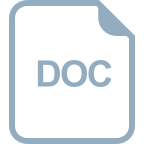
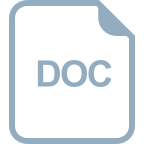
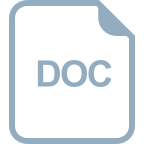
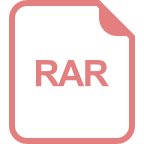














