51单片机使用dht11温度湿度模块在数码管上显示
时间: 2024-12-14 19:25:51 浏览: 15
在51单片机中使用DHT11温度湿度模块并将数据展示到数码管上,一般步骤如下:
1. **硬件连接**:
- 将DHT11的VCC连接到单片机的+5V电源,数据线(D4)连接到单片机的IO口(通常是P0或P1)。地线(GND)也连接在一起。
- 数码管连接到单片机的7段显示接口,根据具体的数码管型号配置驱动电路。
2. **软件编程**:
a. **DHT11数据读取**:先调用之前编写的DHT11读取函数,如`dht11_read()`,获取温度和湿度数据。
b. **数据处理**:从返回的`DHT11Data`结构体中提取温度和湿度值。由于数码管只能显示数字,所以可能需要将小数部分舍去或转换为整数形式。
c. **数码管显示**:根据处理后的数值,使用对应的ASCII字符编码映射到7段显示模式,然后控制每个数码管的段选信号和字形码。
```c
for (int i = 0; i <= 9; i++) {
if (temp / 10 == i || hum % 10 == i) {
// 设置对应数码管显示相应位
// 数码管的显示逻辑取决于具体硬件和编程模型
}
}
```
3. **循环更新**:为了实时显示温度和湿度,可以在循环中定期调用数据读取和显示函数。
相关问题
2,项目题目 《无线温湿度报警系统》 项目功能: ①获取温湿度数据 ②. 设置温湿度数据阀值,低于或超过阀值则进行系统报警(蜂鸣器、LED) 3. 使用OLED显示屏显示温湿度数据和系统状态 4 通过串口通信方式,将温湿度数据和系统状态发送到用户端(USB线连接PC、蓝牙连接手机) ⑤ 系统报警状态下,通过一定方式进行自调整(通风,加热,加湿) STM32读取DHT11温湿度传感器并显示在数码管上 使用材料:STM32F103、DHT11温湿度传感器、OLED 平台:keil 技术实现:利用单片机读DHT11的温湿度数据,并且显示到OLED上 ,求代码
由于这是一个比较复杂的项目,需要多个模块的代码来实现。以下是基本框架和代码,供参考:
主函数:
```c
#include "stm32f10x.h"
#include "dht11.h"
#include "oled.h"
#include "usart.h"
#include "delay.h"
int main(void) {
uint8_t temp, humi;
int alarm = 0;
int fan = 0, heater = 0, humidifier = 0;
int count = 0;
SystemInit();
OLED_Init();
USART1_Init(115200);
DHT11_Init();
while (1) {
DHT11_Read_Data(&temp, &humi);
OLED_Show_Temperature_Humidity(temp, humi);
if (temp < TEMP_LOW_THRESHOLD || temp > TEMP_HIGH_THRESHOLD ||
humi < HUMI_LOW_THRESHOLD || humi > HUMI_HIGH_THRESHOLD) {
alarm = 1;
GPIO_SetBits(GPIOA, GPIO_Pin_8);
GPIO_SetBits(GPIOC, GPIO_Pin_13);
} else {
alarm = 0;
GPIO_ResetBits(GPIOA, GPIO_Pin_8);
GPIO_ResetBits(GPIOC, GPIO_Pin_13);
}
if (alarm) {
if (count < 10) {
fan = 1;
heater = 1;
humidifier = 1;
} else {
fan = 0;
heater = 0;
humidifier = 0;
count = 0;
}
count++;
}
USART_SendData(USART1, temp);
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
USART_SendData(USART1, humi);
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
USART_SendData(USART1, alarm);
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
USART_SendData(USART1, fan);
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
USART_SendData(USART1, heater);
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
USART_SendData(USART1, humidifier);
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
delay_ms(1000);
}
}
```
DHT11模块:
```c
#include "dht11.h"
#define DHT11_GPIO GPIOB
#define DHT11_Pin GPIO_Pin_2
void DHT11_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = DHT11_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_GPIO, &GPIO_InitStructure);
}
void DHT11_Set_Input(void) {
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_GPIO, &GPIO_InitStructure);
}
void DHT11_Set_Output(void) {
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_GPIO, &GPIO_InitStructure);
}
uint8_t DHT11_Read_Byte(void) {
uint8_t byte = 0;
for (int i = 0; i < 8; i++) {
byte <<= 1;
while (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_Pin) == RESET);
delay_us(28);
if (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_Pin) == SET) {
byte |= 0x01;
}
while (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_Pin) == SET);
}
return byte;
}
void DHT11_Read_Data(uint8_t* temp, uint8_t* humi) {
uint8_t data[5];
DHT11_Set_Output();
GPIO_ResetBits(DHT11_GPIO, DHT11_Pin);
delay_ms(18);
GPIO_SetBits(DHT11_GPIO, DHT11_Pin);
delay_us(30);
DHT11_Set_Input();
while (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_Pin) == SET);
while (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_Pin) == RESET);
while (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_Pin) == SET);
for (int i = 0; i < 5; i++) {
data[i] = DHT11_Read_Byte();
}
if ((data[0] + data[1] + data[2] + data[3]) == data[4]) {
*humi = data[0];
*temp = data[2];
}
}
```
OLED模块:
```c
#include "oled.h"
#define OLED_GPIO GPIOA
#define OLED_DC GPIO_Pin_2
#define OLED_RST GPIO_Pin_3
#define OLED_CS GPIO_Pin_4
void OLED_Write_Command(uint8_t cmd) {
GPIO_ResetBits(OLED_GPIO, OLED_DC);
GPIO_ResetBits(OLED_GPIO, OLED_CS);
SPI_I2S_SendData(SPI1, cmd);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_BSY) == SET);
GPIO_SetBits(OLED_GPIO, OLED_CS);
}
void OLED_Write_Data(uint8_t data) {
GPIO_SetBits(OLED_GPIO, OLED_DC);
GPIO_ResetBits(OLED_GPIO, OLED_CS);
SPI_I2S_SendData(SPI1, data);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_BSY) == SET);
GPIO_SetBits(OLED_GPIO, OLED_CS);
}
void OLED_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = OLED_DC | OLED_RST | OLED_CS;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(OLED_GPIO, &GPIO_InitStructure);
SPI_InitTypeDef SPI_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1, ENABLE);
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_4;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_Init(SPI1, &SPI_InitStructure);
SPI_Cmd(SPI1, ENABLE);
OLED_Write_Command(0xAE); //display off
OLED_Write_Command(0x20); //Set Memory Addressing Mode
OLED_Write_Command(0x10); //00,Horizontal Addressing Mode;01,Vertical Addressing Mode;10,Page Addressing Mode (RESET);11,Invalid
OLED_Write_Command(0xb0); //Set Page Start Address for Page Addressing Mode,0-7
OLED_Write_Command(0xc8); //Set COM Output Scan Direction
OLED_Write_Command(0x00); //---set low column address
OLED_Write_Command(0x10); //---set high column address
OLED_Write_Command(0x40); //--set start line address
OLED_Write_Command(0x81); //--set contrast control register
OLED_Write_Command(0xff);
OLED_Write_Command(0xa1); //--set segment re-map 0 to 127
OLED_Write_Command(0xa6); //--set normal display
OLED_Write_Command(0xa8); //--set multiplex ratio(1 to 64)
OLED_Write_Command(0x3f); //
OLED_Write_Command(0xa4); //0xa4,Output follows RAM content;0xa5,Output ignores RAM content
OLED_Write_Command(0xd3); //-set display offset
OLED_Write_Command(0x00); //-not offset
OLED_Write_Command(0xd5); //--set display clock divide ratio/oscillator frequency
OLED_Write_Command(0xf0); //--set divide ratio
OLED_Write_Command(0xd9); //--set pre-charge period
OLED_Write_Command(0x22); //
OLED_Write_Command(0xda); //--set com pins hardware configuration
OLED_Write_Command(0x12);
OLED_Write_Command(0xdb); //--set vcomh
OLED_Write_Command(0x20); //0x20,0.77xVcc
OLED_Write_Command(0x8d); //--set DC-DC enable
OLED_Write_Command(0x14); //
OLED_Write_Command(0xaf); //--turn on oled panel
OLED_Clear();
}
void OLED_Clear(void) {
OLED_Write_Command(0xb0);
OLED_Write_Command(0x00);
OLED_Write_Command(0x10);
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 128; j++) {
OLED_Write_Data(0x00);
}
}
}
void OLED_Show_Char(uint8_t x, uint8_t y, uint8_t chr) {
if (x > 127 || y > 7) {
return;
}
uint8_t c = chr - ' ';
OLED_Write_Command(0xb0 + y);
OLED_Write_Command(x & 0x0f);
OLED_Write_Command(0x10 | ((x >> 4) & 0x0f));
for (int i = 0; i < 6; i++) {
OLED_Write_Data(Font6x8[c][i]);
}
}
void OLED_Show_String(uint8_t x, uint8_t y, char* str) {
while (*str) {
OLED_Show_Char(x, y, *str++);
x += 6;
}
}
void OLED_Show_Temperature_Humidity(uint8_t temp, uint8_t humi) {
OLED_Clear();
OLED_Show_String(0, 0, "TEMP:");
OLED_Show_Char(48, 0, temp / 10 + '0');
OLED_Show_Char(54, 0, temp % 10 + '0');
OLED_Show_Char(60, 0, 'C');
OLED_Show_String(0, 1, "HUMI:");
OLED_Show_Char(48, 1, humi / 10 + '0');
OLED_Show_Char(54, 1, humi % 10 + '0');
OLED_Show_Char(60, 1, '%');
}
```
USART模块:
```c
#include "usart.h"
void USART1_Init(uint32_t baud_rate) {
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = baud_rate;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
int USART_SendData(USART_TypeDef* usart, uint8_t data) {
while (USART_GetFlagStatus(usart, USART_FLAG_TXE) == RESET);
USART_SendData(usart, data);
return data;
}
uint8_t USART_ReceiveData(USART_TypeDef* usart) {
while (USART_GetFlagStatus(usart, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(usart);
}
```
其他模块:
delay.h:
```c
#ifndef __DELAY_H
#define __DELAY_H
#include "stm32f10x.h"
void delay_us(uint32_t us);
void delay_ms(uint32_t ms);
#endif
```
delay.c:
```c
#include "delay.h"
static uint32_t us_ticks = 0;
void SysTick_Handler(void) {
if (us_ticks != 0) {
us_ticks--;
}
}
void delay_us(uint32_t us) {
us_ticks = us;
while (us_ticks);
}
void delay_ms(uint32_t ms) {
while (ms--) {
delay_us(1000);
}
}
```
Font6x8.h:
```c
#ifndef __FONT6X8_H
#define __FONT6X8_H
#include "stm32f10x.h"
extern const uint8_t Font6x8[][6];
#endif
```
Font6x8.c:
```c
#include "Font6x8.h"
const uint8_t Font6x8[][6] = {
{0x00,0x00,0x00,0x00,0x00,0x00},/*" ",0*/
{0x00,0x00,0x5f,0x00,0x00,0x00},/*"!",1*/
{0x00,0x07,0x00,0x07,0x00,0x00},/*""",2*/
{0x14,0x7f,0x14,0x7f,0x14,0x00},/*"#",3*/
{0x24,0x2a,0x7f,0x2a,0x12,0x00},/*"$",4*/
{0x23,0x13,0x08,0x64,0x62,0x00},/*"%",5*/
{0x36,0x49,0x55,0x22,0x50,0x00},/*"&",6*/
{0x00,0x05,0x03,0x00,0x00,0x00},/*"'",7*/
{0x00,0x1c,0x22,0x41,0x00,0x00},/*"(",8*/
{0x00,0x41,0x22,0x1c,0x00,0x00},/*")",9*/
{0x14,0x08,0x3e,0x08,0x14,0x00},/*"*",10*/
{0x08,0x08,0x3e,0x08,0x08,0x00},/*"+",11*/
{0x00,0x50,0x30,0x00,0x00,0x00},/*",",12*/
{0x08,0x08,0x08,0x08,0x08,0x00},/*"-",13*/
{0x00,0x60,0x60,0x00,0x00,0x00},/*".",14*/
{0x20,0x10,0x08,0x04,0x02,0x00},/*"/",15*/
{0x3e,0x51,0x49,0x45,0x3e,0x00},/*"0",16*/
{0x00,0x42,0x7f,0x40,0x00,0x00},/*"1",17*/
{0x42,0x61,0x51,0x49,0x46,0x00},/*"2",18*/
{0x21,0x41,0x45,0x4b,0x31,0x00},/*"3",19*/
{0x18,0x14,0x12,0x7f,0x10,0x00},/*"4",20*/
{0x27,0x45,0x45,0x45,0x39,0x00},/*"5",21*/
{0x3c,0x4a,0x49,0x49,0x30,0x00},/*"6",22*/
{0x01,0x71,0x09,0x05,0x03,0x00},/*"7",23*/
如何设计一个基于51单片机的温湿度控制系统,并实现数码管显示和独立按键设置报警阈值?
设计一个基于51单片机的温湿度控制系统,首先需要理解项目的各个组成部分及其功能。首先,DHT11传感器负责采集环境中的温度和湿度数据。51单片机作为系统核心,负责处理传感器数据、控制数码管显示以及响应按键输入来设定报警阈值。
参考资源链接:[51单片机温湿度控制系统设计与仿真](https://wenku.csdn.net/doc/13bisfrt8j?spm=1055.2569.3001.10343)
在硬件连接方面,需将DHT11的数据线连接到单片机的某个I/O端口,并确保其电源和地线正确连接。数码管的各个段同样连接到单片机的I/O端口,并通过控制各个段的高低电平来显示不同的数字和字符。独立按键需要连接到单片机的另一个I/O端口,并通过编写相应的中断服务程序或轮询程序来检测按键状态。
软件编程是实现系统功能的关键,包括初始化单片机的各种外设,比如定时器、中断系统、I/O端口等。编程时需要编写函数来读取DHT11传感器的数据,并将读取到的温湿度值通过数码管显示出来。同时,编写按键处理程序来实现报警阈值的设定,并在温湿度超出预设阈值时通过控制继电器或者直接输出高电平信号到报警器上。
实现原理图、流程图和仿真验证也是设计过程的重要部分。可以通过Proteus软件绘制系统的原理图,并设置各个元器件的属性和连接。流程图将帮助你更清晰地梳理程序的逻辑流程。在硬件搭建正确无误后,可以通过Proteus进行仿真测试,确保系统运行稳定且符合预期的功能。
最后,为了方便调试和维护,建议编写源代码时注意代码的结构和注释,以便在实际应用中快速定位问题并进行改进。源代码的模块化和良好的注释也能便于其他开发者理解和使用你的系统。
综上所述,你将能够构建一个基本的温湿度控制系统,并通过仿真软件验证其功能。对于想要更深入了解51单片机应用和系统设计的读者,我推荐参考《51单片机温湿度控制系统设计与仿真》这本书,它不仅详细介绍了本项目的原理图、流程图、源代码等关键资料,还提供了完整的系统设计思路和实践方法,对于学习和研究有着极大的帮助。
参考资源链接:[51单片机温湿度控制系统设计与仿真](https://wenku.csdn.net/doc/13bisfrt8j?spm=1055.2569.3001.10343)
阅读全文
相关推荐
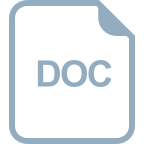
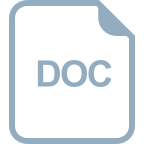
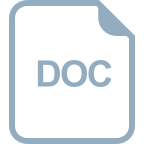
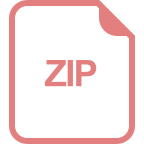
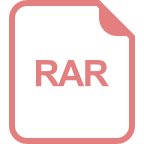
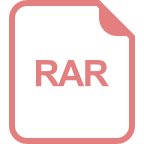
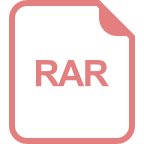
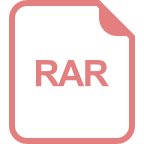
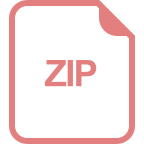
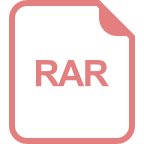
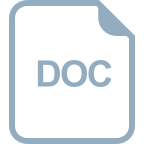
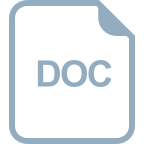
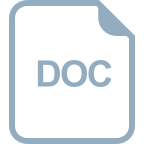
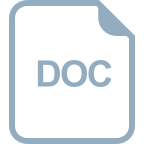
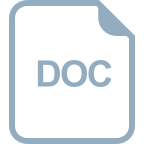
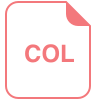