用C++语言获取xml文件中固定内容
时间: 2024-10-12 13:12:05 浏览: 11
在C++中,你可以使用一些第三方库如pugixml、TinyXML或者rapidxml等来解析XML文件并提取特定内容。这里以pugixml为例说明如何操作:
首先,你需要包含pugixml库的头文件,并链接相应的库到你的项目中。安装完成后,可以这样做:
```cpp
#include <pugixml.hpp>
using xmlpp::document;
using xmlpp::node_type;
using xmlpp::attribute;
// 解析XML文件
void extractFixedContent(const std::string& filePath) {
document doc; // 创建文档对象
if (!doc.loadFile(filePath)) { // 尝试加载文件
std::cerr << "Failed to load XML file: " << filePath << std::endl;
return;
}
// 查找固定节点,例如假设我们要查找名为"content"的元素
node_type root = doc.first_child();
for (const auto& child : root.children()) {
if (child.name() == "content") {
attribute attrValue = child.attribute("name"); // 获取属性值,如果存在
if (attrValue) {
std::cout << "Found fixed content: " << attrValue.value() << std::endl;
} else {
std::cout << "Content found without 'name' attribute." << std::endl;
}
}
}
}
```
在这个例子中,我们假设有个`<content name="specific_value">...</content>`的结构,然后通过遍历根节点的子节点来找到这个元素,并获取其"name"属性的值。
阅读全文
相关推荐
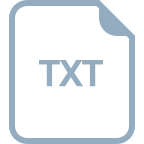
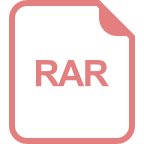
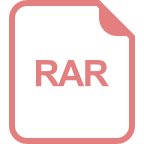















