springboot整合mybatis的模糊查询
时间: 2023-08-21 17:12:14 浏览: 150
在SpringBoot整合Mybatis中进行模糊查询,可以使用Mybatis-Plus提供的查询方法来实现。首先,在实体类中添加需要进行模糊查询的字段,并在字段上添加@TableField注解。然后,在Mapper接口中使用@Select注解定义查询方法,使用@Param注解指定参数名称。在SQL语句中使用LIKE关键字进行模糊匹配,可以使用%作为通配符。最后,在Service层调用Mapper接口的查询方法即可实现模糊查询。例如,假设需要查询名字中包含"张"的用户,可以按照以下步骤进行操作:
1. 在实体类中添加名字字段,并在字段上添加@TableField注解,如下所示:
```java
@TableField(value = "name")
private String name;
```
2. 在Mapper接口中添加查询方法,使用@Select注解定义SQL语句,如下所示:
```java
@Select("SELECT * FROM user WHERE name LIKE CONCAT('%', #{name}, '%')")
List<User> findUsersByName(@Param("name") String name);
```
3. 在Service层调用Mapper接口的查询方法,如下所示:
```java
List<User> users = userMapper.findUsersByName("张");
```
这样就可以实现在SpringBoot整合Mybatis中进行模糊查询了。[1]
相关问题
springboot 整合 mybatis-plus 分页 并查询mapper.xml
好的,下面是 Spring Boot 整合 MyBatis-Plus 进行分页查询的示例代码:
首先,需要在 pom.xml 中添加 MyBatis-Plus 和 MySQL 的依赖:
```xml
<dependencies>
<!-- MyBatis-Plus -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.1</version>
</dependency>
<!-- MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
</dependencies>
```
然后,需要在 application.properties 中配置 MySQL 数据库连接信息:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
```
接下来,创建一个实体类和对应的 Mapper 接口:
```java
@Data
public class User {
private Long id;
private String name;
private Integer age;
private String email;
}
@Mapper
public interface UserMapper extends BaseMapper<User> {
IPage<User> selectUserPage(Page<User> page, @Param("name") String name);
}
```
其中,User 类是实体类,UserMapper 是 Mapper 接口,继承了 MyBatis-Plus 的 BaseMapper 接口。
注意,在 UserMapper 接口中,我们定义了一个名为 selectUserPage 的方法,它接受一个 Page 对象和一个 name 参数,返回一个 IPage 对象。这个方法将在 Controller 中调用,用于分页查询用户信息。
最后,编写 Controller 类,通过调用 UserMapper 中定义的 selectUserPage 方法来进行分页查询:
```java
@RestController
public class UserController {
@Autowired
private UserMapper userMapper;
@GetMapping("/users")
public IPage<User> getUserList(@RequestParam(defaultValue = "1") Integer pageNum,
@RequestParam(defaultValue = "10") Integer pageSize,
@RequestParam(required = false) String name) {
Page<User> page = new Page<>(pageNum, pageSize);
return userMapper.selectUserPage(page, name);
}
}
```
在 getUserList 方法中,我们首先创建了一个 Page 对象,表示要查询的分页信息。然后,调用 userMapper.selectUserPage 方法来进行分页查询,并将结果返回给前端。
最后,这里提供一个 Mapper.xml 文件的示例,用于实现分页查询:
```xml
<select id="selectUserPage" resultMap="BaseResultMap">
select *
from user
<if test="name != null">
where name like concat('%', #{name}, '%')
</if>
<if test="orderBy != null">
order by ${orderBy}
</if>
</select>
```
其中,selectUserPage 方法的实现与上面的示例代码类似,这里不再赘述。注意,这里使用了 MyBatis 的动态 SQL 功能,根据传入的参数来动态生成 SQL 语句。具体来说,如果传入了 name 参数,则会在 SQL 语句中添加一个 where 子句,用于按照用户名进行模糊查询;如果传入了 orderBy 参数,则会在 SQL 语句中添加一个 order by 子句,用于按照指定字段排序。
mybatis-plus整合springboot模糊查询
在MyBatis-Plus中,我们可以使用LambdaQueryWrapper来进行模糊查询的整合。下面是一个示例代码:
```java
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
@Override
public List<User> searchUser(String keyword) {
LambdaQueryWrapper<User> queryWrapper = new LambdaQueryWrapper<>();
queryWrapper.like(User::getName, keyword);
return baseMapper.selectList(queryWrapper);
}
}
```
在这个例子中,我们假设有一个User实体类,包含一个name属性。在searchUser方法中,我们创建了一个LambdaQueryWrapper对象,并使用like方法来设置模糊查询条件。在这里,我们使用User::getName来指定要查询的属性,然后传入关键字keyword。
然后,我们调用baseMapper的selectList方法来执行查询,并返回结果。
这样,你就可以在MyBatis-Plus整合Spring Boot中进行模糊查询了。
阅读全文
相关推荐
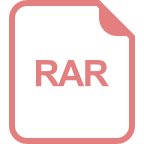
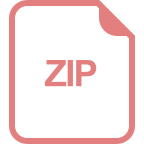
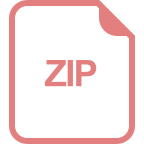
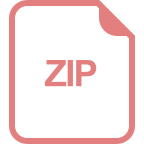
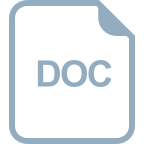
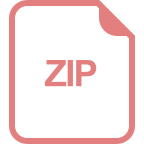
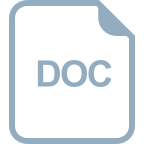

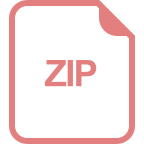
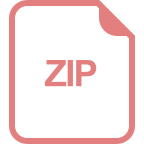
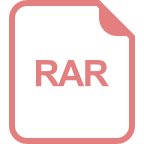
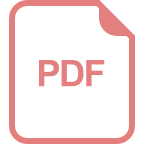
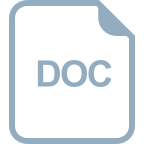
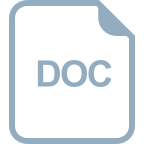