请用【java】帮我帮我写一个PID的速度环的代码
时间: 2024-10-22 10:28:35 浏览: 17
在Java中,实现PID速度控制环的基本代码可能如下所示。这里我们假设有一个模拟的`Sensor`类提供实际速度反馈,一个`Motor`类接受PID输出调整电机速度。请注意,这只是一个基础版本的PID控制器,实际应用中可能需要更复杂的错误处理和参数调整部分:
```java
public class PIDController {
private double kp;
private double ki;
private double kd;
private double integral;
private double derivative;
private long lastErrorTime = 0;
// Constructor to set gains
public PIDController(double kp, double ki, double kd) {
this.kp = kp;
this.ki = ki;
this.kd = kd;
}
// Update method with sensor reading and desired speed
public void update(double desiredSpeed, Sensor sensor) {
double currentTime = System.currentTimeMillis();
double error = desiredSpeed - sensor.getActualSpeed();
// Calculate integral term
integral += (currentTime - lastErrorTime) * error;
if (integral > integralMax) {
integral = integralMax;
} else if (integral < integralMin) {
integral = integralMin;
}
lastErrorTime = currentTime;
// Calculate derivative term (assuming fixed sampling time)
derivative = (error - lastErrorTime);
previousError = error;
// PID output calculation
double output = kp * error + ki * integral + kd * derivative;
// Motor control with PWM signal
motor.setSpeed(output);
}
private double previousError;
private double integralMax, integralMin; // Set these limits as needed
}
// Example usage:
class Main {
public static void main(String[] args) {
PIDController pid = new PIDController(kpValue, kiValue, kdValue);
Sensor sensor = new Sensor(); // Your sensor implementation
Motor motor = new Motor(); // Your motor controller implementation
while (true) {
pid.update(desiredSpeed, sensor);
}
}
}
```
记得替换`kpValue`, `kiValue`, `kdValue`为合适的比例、积分和微分增益值,并根据你的硬件需求配置`integralMax`和`integralMin`。
阅读全文
相关推荐
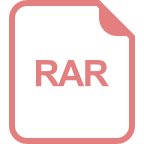
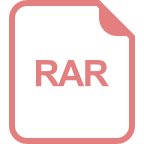
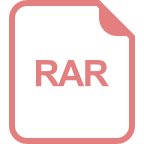
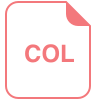
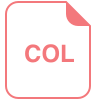
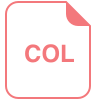
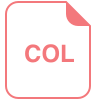
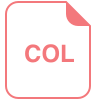
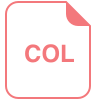
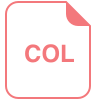
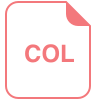
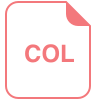
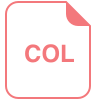
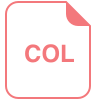
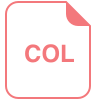
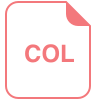
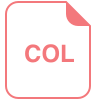
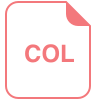
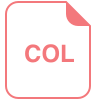