摄影测量后方位Python实现
时间: 2024-04-06 20:26:53 浏览: 112
摄影测量后方位是指通过已知的摄影测量数据,计算出相片的外方位元素,包括相片的旋转角度和平移量。在Python中,可以使用一些库和算法来实现摄影测量后方位的计算。
一种常用的方法是使用OpenCV库进行图像处理和计算。以下是一个简单的摄影测量后方位的Python实现示例:
```python
import cv2
import numpy as np
def calculate_exterior_orientation(image_points, ground_points, camera_matrix):
# 通过图像点和地面点计算外方位元素
# 构建齐次坐标矩阵
image_points_homogeneous = np.hstack((image_points, np.ones((image_points.shape[0], 1))))
ground_points_homogeneous = np.hstack((ground_points, np.ones((ground_points.shape[0], 1))))
# 使用最小二乘法求解外方位元素
A = np.zeros((2 * image_points.shape[0], 12))
for i in range(image_points.shape[0]):
A[2 * i, 0:4] = -ground_points_homogeneous[i]
A[2 * i, 8:12] = image_points_homogeneous[i, 0] * ground_points_homogeneous[i]
A[2 * i + 1, 4:8] = -ground_points_homogeneous[i]
A[2 * i + 1, 8:12] = image_points_homogeneous[i, 1] * ground_points_homogeneous[i]
_, _, V = np.linalg.svd(A)
exterior_orientation = V[-1, :].reshape((3, 4))
# 使用相机内参数矩阵反投影到相机坐标系
camera_matrix_inv = np.linalg.inv(camera_matrix)
exterior_orientation = np.dot(camera_matrix_inv, exterior_orientation)
return exterior_orientation
# 示例数据
image_points = np.array([[100, 200], [300, 400], [500, 600]])
ground_points = np.array([[10, 20, 30], [40, 50, 60], [70, 80, 90]])
camera_matrix = np.array([[1000, 0, 500], [0, 1000, 500], [0, 0, 1]])
# 计算外方位元素
exterior_orientation = calculate_exterior_orientation(image_points, ground_points, camera_matrix)
print(exterior_orientation)
```
这个示例代码使用了OpenCV库中的函数和矩阵运算来实现摄影测量后方位的计算。你可以根据自己的需求和数据进行相应的修改和扩展。
阅读全文
相关推荐
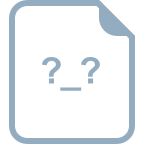
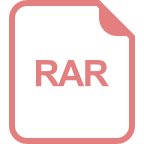
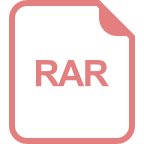
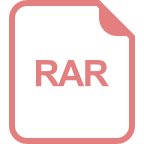
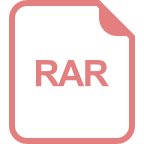
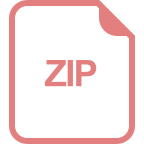
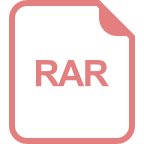
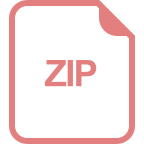
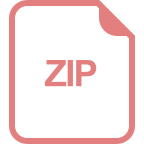
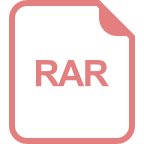
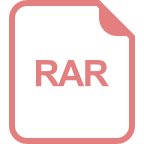
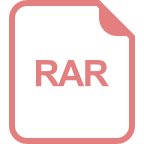
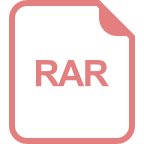
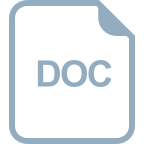
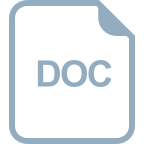
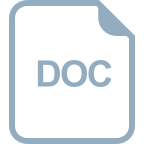
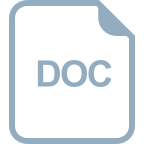