现某学校的学生信息见表所示,我们需要编写Python程序,实现学生 信息的录人与打印
时间: 2024-09-07 11:01:31 浏览: 50
首先,为了实现学生信息的录入与打印功能,我们可以创建一个简单的字典数据结构来存储每个学生的姓名和其它相关信息(比如学号、成绩等)。在Python中,你可以按照以下步骤设计这个程序:
1. 定义一个函数 `input_student_info` 来获取新学生的信息并添加到字典中:
```python
def input_student_info():
name = input("请输入学生姓名:")
student_id = input("请输入学号:")
score = float(input("请输入学习成绩:"))
student_dict[student_id] = {"name": name, "score": score}
```
2. 创建一个空字典 `student_dict` 来存放所有学生的信息:
```python
student_dict = {}
```
3. 编写一个函数 `print_students` 来打印所有学生的信息:
```python
def print_students():
if not student_dict:
print("当前没有任何学生信息.")
else:
for id, details in student_dict.items():
print(f"学号: {id}, 姓名: {details['name']}, 成绩: {details['score']}")
```
4. 提供用户交互,让用户选择是否继续输入或查看已有的学生信息:
```python
while True:
action = input("请选择操作:\n1. 输入学生信息\n2. 查看所有学生信息\n3. 退出\n")
if action == '1':
input_student_info()
elif action == '2':
print_students()
elif action == '3':
break
else:
print("无效的操作,请重新选择.")
```
现在你有了一个基本的Python程序框架,可以根据需要添加错误处理和界面改进。如果你有具体的数据结构需求或者其他功能要求,请告诉我。
阅读全文
相关推荐
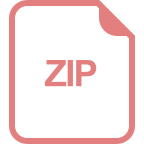
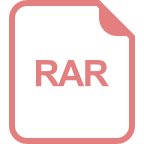
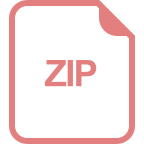
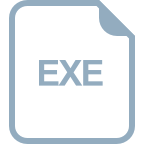
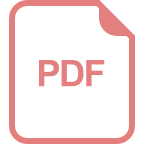
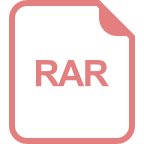
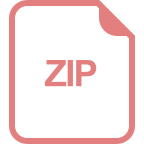
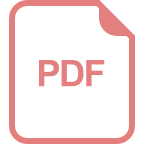
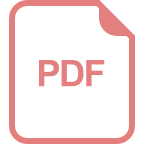
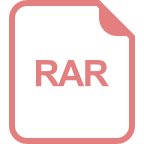
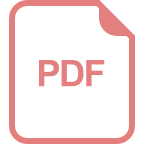
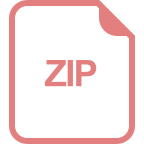