sprngBoot如何将web请求返回的bigdecimal类型数据统一处理成保留两位小数 并使用配置成全局应用
时间: 2024-11-12 14:41:31 浏览: 5
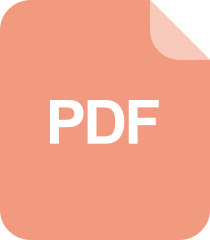
Java保留两位小数的几种写法总结
在Spring Boot中,如果你想要将所有的`BigDecimal`类型的响应结果都转换为保留两位小数,你可以使用Jackson库来进行配置。Jackson默认会提供精确到毫秒的精度,但我们可以定制它以满足你的需求。
首先,在`application.properties`或`application.yml`中找到`spring.jackson.number.format`相关的配置项,但这个配置已经不再推荐使用,因为它的作用有限。现在更推荐使用`ObjectMapper`的配置来全局控制数字序列化的行为。你可以在Spring Boot主类上或者启动类的`@PostConstruct`方法中动态地创建`ObjectMapper`并设置格式:
```java
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class JsonSerializerConfigurer {
@Value("${json.number.format:yyyy-MM-dd'T'HH:mm:ss.SSSXXX}")
private String defaultDateFormat;
private ObjectMapper objectMapper;
@PostConstruct
public void init() {
this.objectMapper = new ObjectMapper();
objectMapper.configure(JsonGenerator.Feature.WRITE_NUMBERS_AS_DECIMALS, true); // 将所有数字序列化为十进制
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL); // 只包含非null值
// 如果你想限制小数位数,可以创建一个插件(DeserializerModifier)来修改BigDecimals
SimpleNumberFormat plugin = new SimpleNumberFormat("0.##");
objectMapper.registerModule(new CustomNumberDeserializingModule(plugin));
}
public ObjectMapper getObjectMapper() {
return objectMapper;
}
private static class SimpleNumberFormat extends NumberFormat {
// 实现NumberFormat接口的方法,并限制小数点后的位数
// ...
@Override
public StringBuffer format(double d, StringBuffer toAppendTo, FieldPosition pos) {
// 格式化double值并保留两位小数
return super.format(d * 100d, toAppendTo, pos);
}
}
private static class CustomNumberDeserializingModule extends SimpleModule {
private final NumberFormat numberFormat;
CustomNumberDeserializingModule(NumberFormat numberFormat) {
super("customBigDecimal");
this.numberFormat = numberFormat;
}
@Override
protected void setupModule(SetupContext context) {
context.setDefaultPrettyPrinter(new CustomIndentedPrettyPrinter());
addDeserializer(BigDecimal.class, new BigDecimalDeserializer(numberFormat));
}
}
// 自定义BigDecimal的Deserializer
private static class BigDecimalDeserializer extends StdDeserializer<BigDecimal> {
private static final long serialVersionUID = 1L;
private final NumberFormat formatter;
public BigDecimalDeserializer(NumberFormat formatter) {
super(BigDecimal.class);
this.formatter = formatter;
}
@Override
public BigDecimal deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
// 解析出的大数值乘以100并格式化为两位小数
String valueStr = p.getText().trim();
try {
double value = Double.parseDouble(valueStr);
return new BigDecimal(value * 100, context.getConfig().numberFactory).setScale(2, RoundingMode.HALF_UP);
} catch (NumberFormatException e) {
throw ctxt.wrongType(p, valueStr, BigDecimal.class);
}
}
}
}
```
这样,每次处理HTTP请求的响应时,`BigDecimal`类型的值都会被自动转换为保留两位小数的字符串。
阅读全文
相关推荐
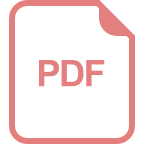
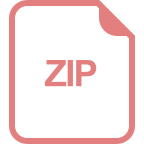















