springboot如何下载另一台ip地址为666.6.66.666主机上的文件到本地,文件具体路径是\\666.6.66.666\e$\共享软件\ymy\123.xls
时间: 2024-10-18 07:23:28 浏览: 19
Spring Boot本身是一个应用框架,并不具备直接操作网络文件的功能。不过,你可以利用其整合的第三方库如Apache HttpClient或者Spring WebClient来实现这个需求。以下是一种基本思路:
首先,你需要添加相关的依赖到你的`pom.xml`或`build.gradle`文件中(如果你使用的是Gradle):
```xml
<!-- Maven -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.x.y</version>
</dependency>
<!-- Gradle (如果使用Spring Bootstarter Web) -->
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'com.squareup.okhttp3:okhttp:4.x.y' // 或者使用OkHttp代替HttpClient
```
然后,你可以创建一个Service或者Repository类,编写一个下载文件的方法,例如:
```java
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
public class FileDownloader {
private static final String FILE_URL = "http://666.6.66.666/e$/共享软件/ymy/123.xls";
public void downloadFile() throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
try {
HttpGet httpGet = new HttpGet(FILE_URL);
CloseableHttpResponse response = httpClient.execute(httpGet);
int responseCode = response.getStatusLine().getStatusCode();
if (responseCode == 200) {
HttpEntity entity = response.getEntity();
saveToFile(entity);
} else {
System.out.println("Failed to download file, HTTP status code: " + responseCode);
}
} finally {
httpClient.close();
}
}
private void saveToFile(HttpEntity entity) throws IOException {
File destinationFile = new File("path/to/save/123.xls");
entity.writeTo(destinationFile);
}
}
```
请注意替换`path/to/save/123.xls`为你实际的本地保存路径。
在这个例子中,我们假设服务器返回了HTTP状态码200,表示请求成功。如果不是这种情况,你可能需要处理异常。
阅读全文
相关推荐
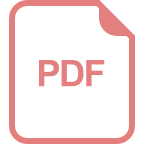
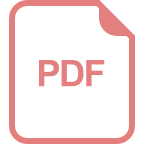
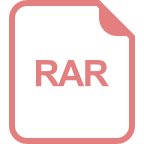















