编写Python函数接收字符串,将字符串全部转换小写,去除所以元音字母
时间: 2024-10-20 16:11:02 浏览: 23
在Python中,你可以编写一个函数来完成这个任务。首先,我们需要创建一个函数`remove_vowels_and_lowercase`,它接受一个字符串作为输入,然后将其转换为小写并移除所有的元音字母。以下是函数的一个实现:
```python
def remove_vowels_and_lowercase(input_string):
vowels = "aeiouAEIOU"
result = ""
for char in input_string.lower():
if char not in vowels:
result += char
return result
# 示例
input_str = "Hello, World!"
output_str = remove_vowels_and_lowercase(input_str)
print(f"原始字符串:{input_str}")
print(f"处理后的字符串:{output_str}")
```
在这个函数中,我们首先将输入字符串转换为小写,然后遍历每个字符。如果字符不是元音字母(不在`vowels`列表内),我们就添加到结果字符串中。最后返回处理后的字符串。
相关问题
用Python实现输入一个以换行结束的字符串,统计并输出字符串中小写元音字母的个数。
好的,可以使用Python的字符串函数和循环语句来实现该功能。以下是示例代码:
```python
# 读取输入的字符串
string = input("请输入一个字符串,以换行结束:")
# 定义小写元音字母
vowels = ['a', 'e', 'i', 'o', 'u']
# 统计小写元音字母的个数
count = 0
for char in string:
if char.lower() in vowels:
count += 1
# 输出结果
print("字符串中小写元音字母的个数为:", count)
```
输入示例:
```
请输入一个字符串,以换行结束:
Hello, world!
```
输出示例:
```
字符串中小写元音字母的个数为: 2
```
在这个例子中,我们首先使用`input()`函数读取用户输入的字符串,然后使用一个包含小写元音字母的列表来定义需要统计的字符集合。接着,我们使用一个循环遍历字符串中的每个字符,并检查该字符是否属于小写元音字母,如果是,则将计数变量加1。最后,我们输出计数结果。
python字符串元音大小写
Python 中的字符串元音是指字母 'a', 'e', 'i', 'o', 'u', 和它们的大写形式 'A', 'E', 'I', 'O', 'U'。你可以通过简单的遍历或使用正则表达式来检查一个字符串中的元音字母,无论是大写还是小写。
例如,你可以创建一个函数来统计字符串中的元音数量:
```python
def count_vowels(s):
vowels = "aeiouAEIOU"
return sum(1 for char in s if char in vowels)
# 使用示例
s = "Hello, World!"
print(count_vowels(s)) # 输出:3
```
如果你需要将所有元音转换为大写或小写,可以这样做:
```python
s = "Hello, World!"
lowercase_vowels = s.lower() # 将所有元音转为小写
uppercase_vowels = s.upper() # 将所有元音转为大写
# 输出:
print(lowercase_vowels) # 输出:"hello, world!"
print(uppercase_vowels) # 输出:"HELLO, WORLD!"
```
阅读全文
相关推荐
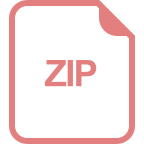
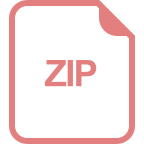
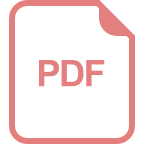













