QT c语言 文件读取10GB 文件 qbytearray接收, 如果文件读取大于2GB 就将文件以2GB一个文件大小保存 写出程序
时间: 2024-10-09 11:05:45 浏览: 54
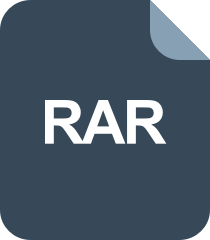
QT写入文件与读取文件内容

在Qt中使用C++编写这样的程序涉及到大型文件的分段读取和处理。由于QT本身不直接支持超过2GB单文件操作,所以我们需要借助于一些策略来处理这种情况。这里是一个简化版的示例,使用了`QFile`、`QByteArray`以及`std::vector<quint64>`来存储每个部分的文件内容:
```cpp
#include <QObject>
#include <QFile>
#include <QByteArray>
#include <vector>
class LargeFileHandler : public QObject
{
public:
LargeFileHandler(const QString& filePath)
: m_filePath(filePath), m_currentSize(0), m_maxFileSize((1 << 31) - 1)
{}
void processFile()
{
QFile file(m_filePath, QIODevice::ReadOnly);
if (!file.open(QIODevice::ReadOnly)) {
qCritical() << "Failed to open the file: " << file.errorString();
return;
}
QByteArray buffer;
while (!file.atEnd()) {
int bytesToRead = qMin(file.bytesAvailable(), m_maxFileSize);
file.read(&buffer[0], bytesToRead);
handleChunk(buffer.data(), bytesToRead);
m_currentSize += bytesToRead;
if (m_currentSize >= m_maxFileSize) {
saveCurrentChunk();
m_currentSize = 0;
buffer.clear();
}
}
if (m_currentSize > 0) { // Process any remaining data
saveCurrentChunk();
}
file.close();
}
private:
void handleChunk(char* data, qint64 size)
{
// 在此处处理每个小块,如解析、存储等操作
// ...
emit chunkProcessed(size);
}
void saveCurrentChunk()
{
QByteArray chunk = QByteArray(data, m_currentSize);
// 实现将chunk写入新的文件或存储系统,这里仅作简单说明
QString newFilePath = m_filePath + "_part_" + QByteArray::number(chunk.size());
QFile outputFile(newFilePath, QIODevice::WriteOnly);
outputFile.write(chunk);
outputFile.close();
}
QString m_filePath;
qint64 m_currentSize;
qint64 m_maxFileSize;
};
// 使用该类的示例:
LargeFileHandler handler("large_file.txt");
QObject::connect(&handler, &LargeFileHandler::chunkProcessed, [] (qint64 size) { /* Handle each chunk processed */ });
handler.processFile();
```
注意,这个示例只是一个基础框架,实际应用中你需要实现`handleChunk`函数来处理每个读取的小块,同时可能还需要处理错误情况并可能添加进度报告等。另外,对于非常大的文件,你可能还会考虑使用更专业的文件系统API,如Linux的`preadv`等。
阅读全文
相关推荐
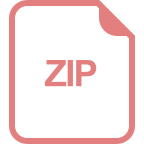
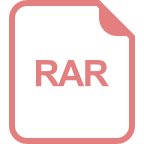


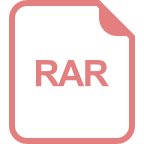
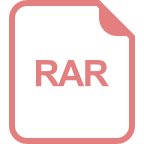
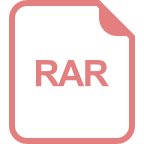
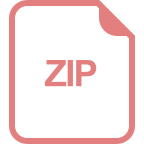
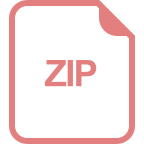
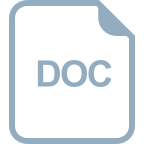
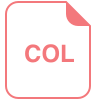
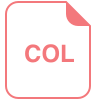



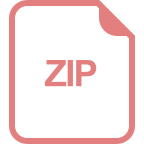
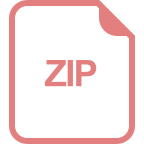
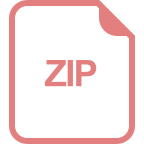