如何用C++编写一个简单的命令行版本的石头、剪刀、布游戏?
时间: 2024-12-05 07:16:15 浏览: 21
在C++中,编写一个简单的命令行版石头、剪刀、布游戏的基本步骤如下:
1. 首先,包括必要的头文件并定义枚举类型来表示游戏选项(石头、剪刀、布)。
```cpp
#include <iostream>
#include <ctime>
using namespace std;
enum GameOptions { ROCK, PAPER, SCISSORS };
```
2. 定义函数让用户输入他们的选择,并将输入转化为GameOptions类型。
```cpp
GameOptions getUserChoice() {
string choice;
cout << "Enter your choice (rock, paper, scissors): ";
cin >> choice;
if (choice == "rock") return ROCK;
else if (choice == "paper") return PAPER;
else if (choice == "scissors") return SCISSORS;
else {
cout << "Invalid input! Please try again.\n";
return getUserChoice();
}
}
```
3. 编写计算机随机选择的游戏选项。
```cpp
GameOptions getComputerChoice() {
srand(time(0));
int randomNum = rand() % 3 + 1; // 生成1到3之间的随机数,对应选项1-3
switch (randomNum) {
case 1: return ROCK;
case 2: return PAPER;
case 3: return SCISSORS;
default: return ROCK; // 如果发生意外,返回默认值
}
}
```
4. 比较两个选择,确定胜负并输出结果。
```cpp
void playRound(GameOptions userChoice, GameOptions computerChoice) {
if (userChoice == computerChoice) {
cout << "It's a tie!\n";
} else if ((userChoice == ROCK && computerChoice == SCISSORS) ||
(userChoice == PAPER && computerChoice == ROCK) ||
(userChoice == SCISSORS && computerChoice == PAPER)) {
cout << "You win!\n";
} else {
cout << "Computer wins!\n";
}
}
```
5. 主循环,反复询问用户直到退出游戏。
```cpp
int main() {
while (true) {
playRound(getUserChoice(), getComputerChoice());
char continueChoice;
cout << "Do you want to play again? (y/n): ";
cin >> continueChoice;
if (continueChoice != 'y') break;
}
return 0;
}
```
阅读全文
相关推荐
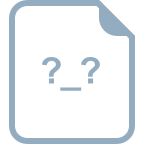
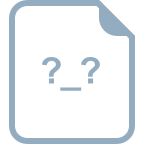
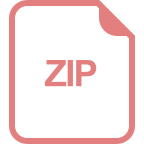
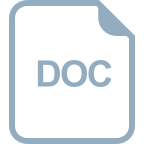

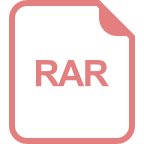
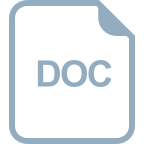
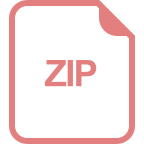
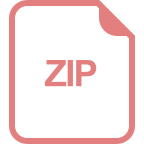
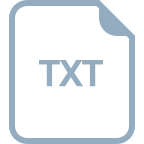
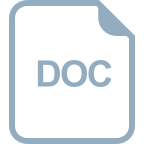
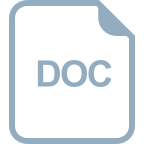
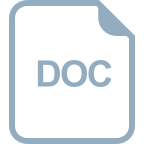
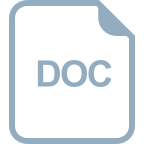
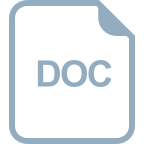


