用启发式算法求解最短路径问题matlab
时间: 2024-12-30 18:17:45 浏览: 5
### 使用A*算法在MATLAB中实现最短路径计算
#### A*算法概述
A*算法是一种启发式搜索算法,能够有效地解决路径规划问题。该算法不仅考虑了从起点到当前节点的实际代价,还加入了估计的剩余距离作为启发式项来指导搜索方向[^1]。
#### MATLAB环境设置
为了在MATLAB环境中实施这一过程,首先需要定义地图模型,即创建一个表示可行区域与障碍物分布情况的数据结构;其次设定起始位置和目标位置坐标;最后编写用于执行A*逻辑的核心程序段落[^2]。
#### 地图建模及参数初始化
假设有一个简单的二值化栅格地图`mapMatrix`,其中0代表可通行空间而1则标记不可逾越之处(如墙壁)。同时指定起点`sPoint=[sx,sy]` 和终点 `ePoint=[ex,ey]` 的具体数值:
```matlab
% 创建一个示例性的8x8大小的地图矩阵(可以根据实际情况调整尺寸)
mapSize = [8 8];
mapMatrix = zeros(mapSize);
% 设置一些随机障碍物的位置 (这里仅作示意用途)
obstaclePositions = [
2 3; % 障碍物位于第2行第3列...
4 7;
6 5];
for i=1:size(obstaclePositions,1)
mapMatrix(sub2ind(mapSize, obstaclePositions(i,1), ...
obstaclePositions(i,2))) = 1;
end
sPoint = [1 1]; % 起点设为左下角第一个单元格中心处
ePoint = [8 8]; % 终点放在右上角最后一个方格内侧中间部位
```
#### 启发函数的设计
对于每一个待考察结点n而言,其f(n)=g(n)+h(n),这里的g(n)是从初始状态到达此节点所累积的成本开销,而h(n)则是预估由当前位置前往最终目的地所需耗费的大致量度。通常情况下可以选择曼哈顿距离或欧几里得距离作为估算依据之一[^3]。
```matlab
function hValue = heuristicFunction(currentNodePosition,endNodePosition)
% 计算两个坐标的直线距离作为初步评估标准
dx = abs(endNodePosition(1)-currentNodePosition(1));
dy = abs(endNodePosition(2)-currentNodePosition(2));
% 这里采用的是棋盘距离/切比雪夫距离(Chebyshev distance)
hValue = max(dx,dy);
end
```
#### 主要流程控制
接下来就是按照既定策略遍历整个解空间直至找到最优解答为止。这期间涉及到优先队列维护、邻域探测等一系列关键技术环节[^4]。
```matlab
openList = {}; % 存储尚未处理过的候选节点列表
closedList = []; % 已经访问过并排除在外的选择集合
pathFoundFlag = false;
% 将出发地点加入开放表头并将之视为首个被探索对象
[~,startIdx]=min([sum((repmat(sPoint,size(mapMatrix(:)),1)-find(~mapMatrix)).^2,[],2)]);
openList{1}={struct('pos',num2cell(sPoint),'costSoFar',0,'estimatedTotalCost',...
sPoint==ePoint ? 0 : heuristicFunction(sPoint,ePoint))};
while ~isempty(openList)&&~pathFoundFlag
[~,idxToExpand]=sort(cellfun(@(nodeData) nodeData.estimatedTotalCost,...
openList)); idxToExpand=idxToExpand(1);
currentNode=openList{idxToExpand};
delete(openList,idxToExpand); clear idxToExpand
if all(strcmp({currentNode.pos{:}},num2cell(ePoint)))
pathFoundFlag=true;
break;
end
closedList=[closedList ; struct('pos',{currentNode.pos})];
neighbors=getNeighbors(currentNode,mapMatrix,closedList);
for eachNeighbor=neighbors'
newGScore=currentNode.costSoFar+getMovementCost(eachNeighbor.mapPos,currentNode.pos);
existingEntry=find(arrayfun(@(entry) isequal(entry.pos,{eachNeighbor.mapPos}),openList),1,'first');
if isempty(existingEntry)||newGScore<openList{existingEntry}.costSoFar
neighborStruct=struct('pos',{eachNeighbor.mapPos},'parentIndex',length(closedList),...
'costSoFar',newGScore,'estimatedTotalCost',...
newGScore+(isequal(num2cell(eachNeighbor.mapPos),num2cell(ePoint))?0:...
heuristicFunction(eachNeighbor.mapPos,ePoint)));
if ~isempty(existingEntry)
openList{existingEntry}=neighborStruct;
else
openList{end+1}=neighborStruct;
end
sort(openList,@(a,b)a.estimatedTotalCost<b.estimatedTotalCost);
end
end
end
```
上述代码片段展示了完整的A*寻径机制框架,当然实际应用时还需要补充诸如获取邻居节点(`getNeighbors`)以及移动成本计算(`getMovementCost`)等功能模块的具体实现细节.
阅读全文
相关推荐













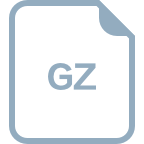
