opencv和yolov8结合
时间: 2023-09-30 22:03:49 浏览: 76
OpenCV和YOLOv8是两个不同的计算机视觉库和模型。OpenCV是一个广泛应用于图像处理和计算机视觉任务的开源库,而YOLOv8是一种目标检测模型,可用于实时物体检测。
要将OpenCV和YOLOv8结合使用,您可以按照以下步骤进行操作:
1. 首先,确保您已经安装了OpenCV和YOLOv8。您可以通过官方网站或其他途径获取它们的安装指南。
2. 使用OpenCV加载图像或视频,并将其传递给YOLOv8模型进行目标检测。您可以使用OpenCV的`cv2.imread()`函数加载图像,或使用`cv2.VideoCapture()`函数捕获视频流。
3. 对于每一帧(图像或视频帧),将其输入到YOLOv8模型中进行目标检测。YOLOv8会返回检测到的物体的边界框和类别信息。
4. 使用OpenCV的绘图函数,例如`cv2.rectangle()`和`cv2.putText()`,将检测到的物体边界框和类别信息绘制在图像上。
5. 可选地,您还可以使用OpenCV的其他功能来进一步处理检测到的物体,例如裁剪、旋转、缩放等。
下面是一个示例代码片段,展示了如何结合OpenCV和YOLOv8进行目标检测:
```python
import cv2
import numpy as np
# Load YOLOv8 model
net = cv2.dnn.readNetFromDarknet("yolov8.cfg", "yolov8.weights")
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# Load image
image = cv2.imread("image.jpg")
# Perform object detection
blob = cv2.dnn.blobFromImage(image, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
# Process detection results
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
# Object detected
center_x = int(detection[0] * image.shape[1])
center_y = int(detection[1] * image.shape[0])
w = int(detection[2] * image.shape[1])
h = int(detection[3] * image.shape[0])
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# Draw bounding boxes and labels
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
font = cv2.FONT_HERSHEY_SIMPLEX
colors = np.random.uniform(0, 255, size=(len(boxes), 3))
for i in range(len(boxes)):
if i in indexes:
x, y, w, h = boxes[i]
label = str(class_ids[i])
color = colors[i]
cv2.rectangle(image, (x, y), (x + w, y + h), color, 2)
cv2.putText(image, label, (x, y - 5), font, 0.5, color, 2)
# Display the result
cv2.imshow("Object Detection", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,上述代码仅为示例,具体的实现细节可能会因您使用的YOLOv8版本和配置而有所不同。要正确运行代码,请确保已正确配置YOLOv8,并将模型文件路径和图像文件路径替换为您自己的路径。
相关推荐
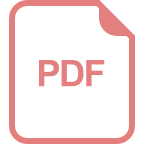
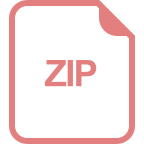
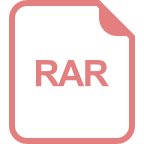














