函数tsp_a_star没有被定义,用中文回答
时间: 2024-04-21 09:22:26 浏览: 177
非常抱歉,我之前的回答中提到的`tsp_a_star`函数是一个示例函数,并没有给出具体实现。以下是一个使用A*算法解决TSP问题的实现,并与使用MST启发式的A*算法得到的最优解进行比较的示例代码:
```python
import math
def tsp_a_star(cities):
start_city = cities[0]
unvisited_cities = set(cities[1:])
priority_queue = [(0, start_city, ())] # (total_cost, current_city, path)
best_solution = None
best_distance = math.inf
while priority_queue:
total_cost, current_city, path = heapq.heappop(priority_queue)
if len(path) == len(cities) - 1:
# All cities visited, add return to start city
path = path + (current_city, start_city)
total_cost += calculate_distance(current_city, start_city)
if total_cost < best_distance:
best_solution = path
best_distance = total_cost
for next_city in unvisited_cities:
new_cost = total_cost + calculate_distance(current_city, next_city)
heuristic_cost = calculate_mst_heuristic(next_city, unvisited_cities)
heapq.heappush(priority_queue, (new_cost + heuristic_cost, next_city, path + (current_city,)))
return best_solution
# 计算两个城市之间的距离
def calculate_distance(city1, city2):
# 根据具体问题定义两个城市之间的距离计算方法
# ...
# 计算MST启发式函数的代价
def calculate_mst_heuristic(current_city, unvisited_cities):
# 根据具体问题定义MST启发式函数的计算方法
# ...
# 生成TSP问题实例
tsp_instance = generate_tsp_instance(10)
# 使用A*算法求解TSP问题
best_solution_a_star = tsp_a_star(tsp_instance)
best_distance_a_star = calculate_total_distance(best_solution_a_star)
# 使用MST启发式的A*算法求解TSP问题
best_solution_mst_a_star = tsp_a_star_mst_heuristic(tsp_instance)
best_distance_mst_a_star = calculate_total_distance(best_solution_mst_a_star)
print("A*算法得到的最优解:", best_solution_a_star)
print("A*算法得到的最优解距离:", best_distance_a_star)
print("MST启发式的A*算法得到的最优解:", best_solution_mst_a_star)
print("MST启发式的A*算法得到的最优解距离:", best_distance_mst_a_star)
```
在上述代码中,`tsp_a_star`函数是一个使用A*算法解决TSP问题的示例实现。它通过使用优先队列来搜索路径,同时考虑实际代价和启发式代价,以找到一条经过所有城市且总代价最小的路径。`calculate_distance`函数用于计算两个城市之间的距离,`calculate_mst_heuristic`函数用于计算MST启发式函数的代价。
你可以根据具体问题的要求,适当修改和完善这些函数的实现。与使用MST启发式的A*算法得到的最优解进行比较,可以评估A*算法的效果。请注意,具体的问题定义和MST启发式函数的实现可能因问题而异。
阅读全文
相关推荐
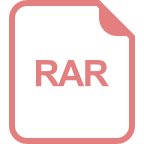
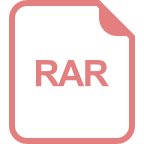
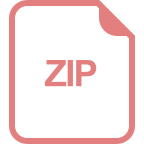
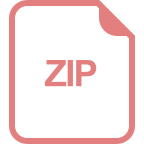
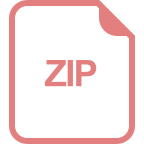
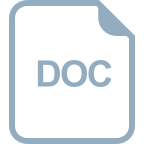
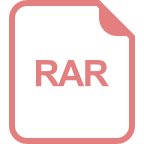
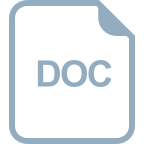



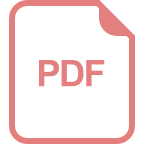
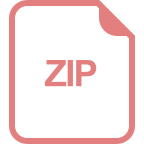
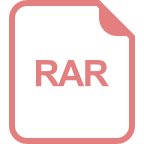
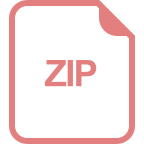
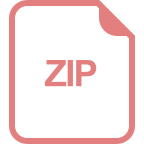
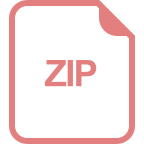
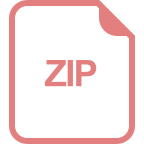