3. 两个羽毛球队进行比赛,各出三人。一队为a,b,c三人,二队为x,y.z三人。已抽签决定比赛名单。有人向队员打听比赛的名单。a说他不和z比,c说他不和x、z比,请编写Java程序找出三队赛手所有可能名单。
时间: 2024-10-14 11:15:05 浏览: 47
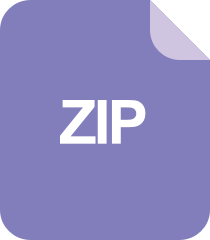
羽毛球队官网_My-profile.zip
这是一个典型的组合排列问题,我们可以用Java的HashSet来存储每个球员不允许对战的对象,然后通过循环来生成所有的可能性。以下是简单的Java代码实现:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
String team1 = "abc";
String team2 = "xyz";
// 创建集合存储不允许对战的人
Set<String> restrictions = new HashSet<>();
restrictions.add("z"); // a不和z比
restrictions.add("x"); // c不和x、z比
// 生成所有可能的比赛阵容
List<List<String>> possibleLineups = generatePossibleLineups(team1, team2, restrictions);
for (List<String> lineup : possibleLineups) {
System.out.println("Team 1: " + lineup.get(0) + ", " + lineup.get(1) + ", " + lineup.get(2));
System.out.println("Team 2: " + lineup.get(3) + ", " + lineup.get(4) + ", " + lineup.get(5));
System.out.println(); // 换行显示下一对
}
}
private static List<List<String>> generatePossibleLineups(String team1, String team2, Set<String> restrictions) {
List<List<String>> result = new ArrayList<>();
permute(team1.toCharArray(), team2.toCharArray(), new char[0], restrictions, result);
return result;
}
private static void permute(char[] arr1, char[] arr2, char[] current, Set<String> restrictions, List<List<String>> result) {
if (current.length == 6) {
result.add(Arrays.asList(new String(current)));
return;
}
for (int i = 0; i < arr1.length && !restrictions.contains(arr1[i] + ""); i++) {
if (!Arrays.stream(current).anyMatch(c -> c == arr1[i])) { // 避免重复
current[current.length] = arr1[i];
permute(arr1, arr2, current, restrictions, result);
// 如果当前元素不在限制列表中,尝试添加到arr2
if (!restrictions.contains(arr2[i] + "")) {
current[current.length] = arr2[i];
permute(arr2, arr1, current, restrictions, result); // 交换arr1和arr2以覆盖所有情况
}
}
}
}
}
```
这个程序会输出所有可能的对阵情况。注意,由于可能出现大量的结果(比如总共有6个人,有三个位置),所以实际运行时可能会比较慢。
阅读全文
相关推荐
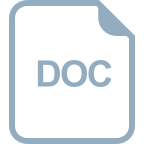
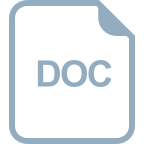
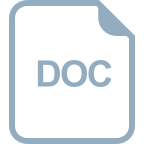
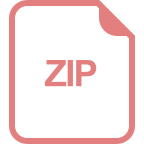
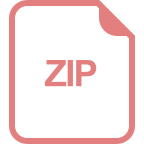
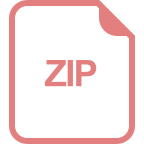
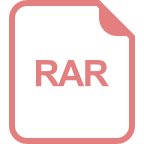
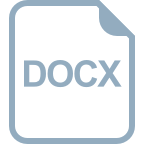
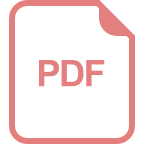
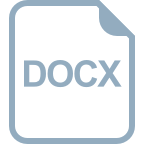
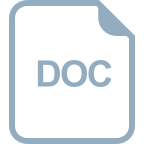
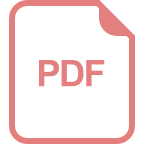
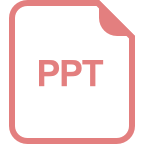
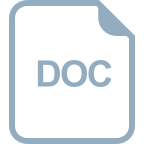
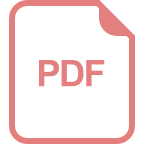
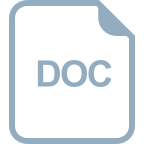
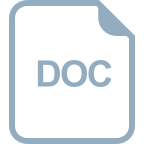