C语言真人互动五子棋
时间: 2025-01-01 21:33:54 浏览: 16
### C语言实现真人互动五子棋游戏
为了创建一个基于C语言的真人互动五子棋游戏,需要考虑几个核心组件:棋盘表示、玩家输入处理以及胜利条件判断。下面是一个简化版本的实现方案。
#### 1. 定义常量和全局变量
定义棋盘大小和其他必要的宏定义来控制程序行为:
```c
#define BOARD_SIZE 15 /* 棋盘尺寸 */
#define EMPTY 0 /* 表示空白位置 */
#define PLAYER_1 1 /* 玩家一标记 */
#define PLAYER_2 2 /* 玩家二标记 */
int board[BOARD_SIZE][BOARD_SIZE]; /* 存储当前棋局状态 */
char player_symbols[] = {' ', 'X', 'O'};/* 显示字符对应关系 */
```
#### 2. 初始化函数
初始化棋盘数据结构并设置初始状态:
```c
void init_board() {
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
board[i][j] = EMPTY;
}
}
}
```
#### 3. 打印棋盘界面
提供直观的方式让用户看到当前的游戏进度:
```c
void print_board() {
printf(" ");
for (int col = 0; col < BOARD_SIZE; ++col) {
printf("%d ", col);
}
printf("\n");
for (int row = 0; row < BOARD_SIZE; ++row) {
printf("%d ", row);
for (int col = 0; col < BOARD_SIZE; ++col) {
char symbol = player_symbols[board[row][col]];
printf("%c ", symbol);
}
printf("\n");
}
}
```
#### 4. 获取用户输入
允许两名玩家轮流下棋,并验证输入的有效性:
```c
bool get_move(int *row, int *col, int current_player) {
while (true) {
printf("Player %d (%c), enter your move as \"row column\": ",
current_player, player_symbols[current_player]);
if (scanf("%d%d", row, col) != 2 || (*row >= BOARD_SIZE || *col >= BOARD_SIZE)) {
printf("Invalid input! Try again.\n");
continue;
}
if (board[*row][*col] != EMPTY) {
printf("This spot is already taken!\n");
continue;
}
break;
}
return true;
}
```
#### 5. 判断胜负逻辑
检查是否有任意一方获胜或平局情况发生:
```c
bool check_winner(int last_row, int last_col, int player_id) {
// Check horizontal/vertical/diagonal lines around the latest piece placed.
const int directions[][2] = {{-1, 0}, {1, 0}, {-1, -1}, {1, 1},
{-1, 1}, {1, -1}, {0, -1}, {0, 1}};
for (const auto& dir : directions) {
int count = 1;
// Count pieces in both positive and negative direction along each line.
for (int d = 0; d < 8 && count < 5; ++d += 7) {
int r = last_row + dir[d / 7];
int c = last_col + dir[(d + 1) / 7];
while (r >= 0 && r < BOARD_SIZE && c >= 0 && c < BOARD_SIZE &&
board[r][c] == player_id) {
++count;
r += dir[d / 7];
c += dir[(d + 1) / 7];
}
}
if (count >= 5) {
return true;
}
}
return false;
}
```
#### 6. 主循环
组合上述功能模块形成完整的交互流程:
```c
#include <stdio.h>
#include <stdbool.h>
// ... 上述所有代码 ...
int main() {
init_board();
bool game_over = false;
int turn = PLAYER_1;
do {
print_board();
int row, col;
get_move(&row, &col, turn);
board[row][col] = turn;
if (check_winner(row, col, turn)) {
print_board();
printf("Congratulations Player %d wins!\n", turn);
game_over = true;
} else {
// Switch turns between players after every valid move.
turn = (turn == PLAYER_1 ? PLAYER_2 : PLAYER_1);
}
} while (!game_over);
return 0;
}
```
此代码片段展示了如何利用基本的数据结构和技术构建简单的两人对弈版五子棋游戏[^1]。当然,在实际应用中还可以加入更多特性如图形化UI支持或是更复杂的AI对手设计等扩展功能。
阅读全文
相关推荐







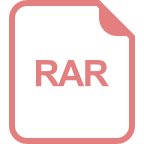


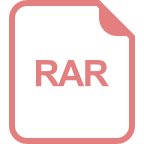

