用c++写一个成绩管理系统
时间: 2024-11-20 15:38:36 浏览: 14
创建一个简单的成绩管理系统可以涉及到几个关键步骤,包括定义学生、课程和成绩等数据结构,并提供相应的操作函数如添加学生、添加课程、记录成绩等。以下是一个简化的C++示例:
```cpp
#include <iostream>
#include <vector>
#include <map>
// 定义学生类
class Student {
public:
std::string name;
int id;
std::map<std::string, int> scores; // 课程ID到分数的映射
void addScore(const std::string& course, int score) {
scores[course] = score;
}
double getAverageScore() const {
if (scores.empty()) return 0;
double total = 0;
for (const auto& score : scores) {
total += score.second;
}
return total / scores.size();
}
};
// 管理系统类
class GradeManagementSystem {
private:
std::vector<Student> students;
public:
void enrollStudent(const std::string& name, int id) {
students.push_back(Student{name, id});
}
void addScoreToStudent(const std::string& studentName, const std::string& course, int score) {
for (auto& student : students) {
if (student.name == studentName) {
student.addScore(course, score);
break;
}
}
}
void printStudentScores(const std::string& studentName) const {
for (const auto& student : students) {
if (student.name == studentName) {
std::cout << "学生: " << student.name << "\n";
std::cout << "平均分: " << student.getAverageScore() << "\n";
break;
}
}
}
};
int main() {
GradeManagementSystem gms;
gms.enrollStudent("张三", 123456);
gms.addScoreToStudent("张三", "数学", 90);
gms.addScoreToStudent("张三", "英语", 85);
gms.printStudentScores("张三");
return 0;
}
```
在这个例子中,我们首先创建了`Student`类表示一个学生,包含姓名、学号以及存储每个课程分数的映射。`GradeManagementSystem`类管理学生列表,提供添加学生、给学生添加成绩以及打印特定学生成绩的功能。
阅读全文
相关推荐
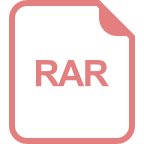



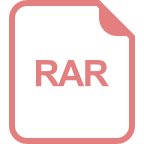
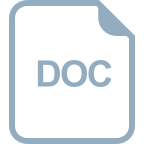
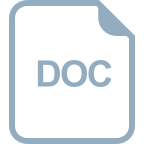
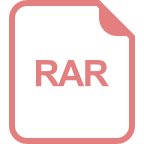

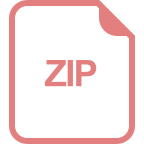
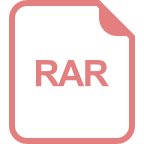



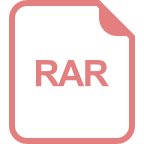

