Java编写程序:单链表操作 1、基本操作: 构造一个空链表 头插法生成链表 尾插法生成链表 往链表中插入一个结点 从结点中删除一个结点 遍历链表
时间: 2024-10-08 14:20:22 浏览: 36
在Java中,单链表是一种基础的数据结构,它由一系列节点组成,每个节点包含数据域和指向下一个节点的引用。以下是关于单链表的基本操作:
1. **构造一个空链表**:
```java
public class LinkedList {
Node head; // head为链表的头节点,初始为空
// 创建一个新的链表表示空列表
public LinkedList() {
head = null;
}
}
```
2. **头插法(Insert at beginning)**:
```java
// 插入新节点到头部
public void insertAt Beginning(int data) {
Node newNode = new Node(data);
newNode.next = head;
head = newNode;
}
```
3. **尾插法(Insert at end)**:
```java
// 在尾部添加新节点
public void insertAtEnd(int data) {
if (head == null) {
head = new Node(data);
return;
}
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = new Node(data);
}
```
4. **往链表中插入一个节点**:
```java
// 在指定位置插入节点(已考虑特殊情况如索引越界)
public void insertNode(int data, int position) {
if (position <= 0) {
insertAtBeginning(data);
} else {
Node newNode = new Node(data);
Node current = head;
for (int i = 0; i < position - 1 && current != null; i++) {
current = current.next;
}
if (current != null) {
newNode.next = current.next;
current.next = newNode;
} else {
System.out.println("Invalid position");
}
}
}
```
5. **从链表中删除一个节点**:
```java
// 删除指定值的节点(如果存在)
public void deleteNode(int value) {
if (head == null) {
return;
}
if (head.data == value) {
head = head.next;
return;
}
Node current = head;
while (current.next != null && current.next.data != value) {
current = current.next;
}
if (current.next != null) {
current.next = current.next.next;
} else {
System.out.println("Value not found in the list.");
}
}
```
6. **遍历链表**:
```java
public void traverseList() {
Node temp = head;
while (temp != null) {
System.out.print(temp.data + " ");
temp = temp.next;
}
System.out.println();
}
```
阅读全文
相关推荐
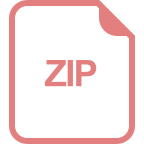
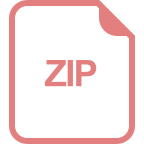
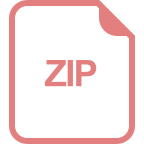
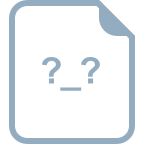
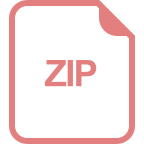
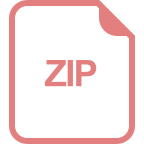
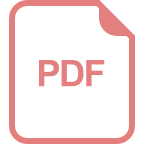
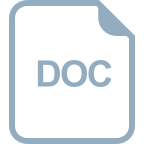
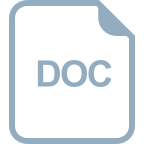
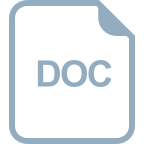