用python生成贪吃蛇小游戏
时间: 2023-07-17 20:01:40 浏览: 34
当然可以!以下是一个简单的Python贪吃蛇小游戏的示例代码:
```python
import pygame
import random
# 游戏窗口大小
WIDTH = 640
HEIGHT = 480
# 蛇身格子大小
GRID_SIZE = 20
# 颜色定义
BLACK = (0, 0, 0)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# 初始化pygame
pygame.init()
window = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("贪吃蛇")
# 定义蛇的类
class Snake:
def __init__(self):
self.body = [(WIDTH / 2, HEIGHT / 2)]
self.direction = random.choice(['up', 'down', 'left', 'right'])
def move(self):
x, y = self.body[0]
if self.direction == 'up':
y -= GRID_SIZE
elif self.direction == 'down':
y += GRID_SIZE
elif self.direction == 'left':
x -= GRID_SIZE
elif self.direction == 'right':
x += GRID_SIZE
self.body.insert(0, (x, y))
self.body.pop()
def change_direction(self, direction):
if direction == 'up' and self.direction != 'down':
self.direction = 'up'
elif direction == 'down' and self.direction != 'up':
self.direction = 'down'
elif direction == 'left' and self.direction != 'right':
self.direction = 'left'
elif direction == 'right' and self.direction != 'left':
self.direction = 'right'
def draw(self):
for x, y in self.body:
pygame.draw.rect(window, GREEN, (x, y, GRID_SIZE, GRID_SIZE))
def grow(self):
x, y = self.body[-1]
if self.direction == 'up':
y += GRID_SIZE
elif self.direction == 'down':
y -= GRID_SIZE
elif self.direction == 'left':
x += GRID_SIZE
elif self.direction == 'right':
x -= GRID_SIZE
self.body.append((x, y))
def check_collision(self):
x, y = self.body[0]
if x < 0 or x >= WIDTH or y < 0 or y >= HEIGHT:
return True
for i in range(1, len(self.body)):
if self.body[i] == self.body[0]:
return True
return False
# 定义食物的类
class Food:
def __init__(self):
self.x = random.randint(0, WIDTH - GRID_SIZE) // GRID_SIZE * GRID_SIZE
self.y = random.randint(0, HEIGHT - GRID_SIZE) // GRID_SIZE * GRID_SIZE
def draw(self):
pygame.draw.rect(window, RED, (self.x, self.y, GRID_SIZE, GRID_SIZE))
# 创建蛇和食物对象
snake = Snake()
food = Food()
# 游戏主循环
running = True
clock = pygame.time.Clock()
while running:
clock.tick(10) # 控制蛇的速度
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake.change_direction('up')
elif event.key == pygame.K_DOWN:
snake.change_direction('down')
elif event.key == pygame.K_LEFT:
snake.change_direction('left')
elif event.key == pygame.K_RIGHT:
snake.change_direction('right')
snake.move()
if snake.body[0] == (food.x, food.y):
snake.grow()
food = Food()
if snake.check_collision():
running = False
window.fill(BLACK)
snake.draw()
food.draw()
pygame.display.flip()
# 游戏结束,退出pygame
pygame.quit()
```
这个示例使用了pygame库来实现游戏窗口、绘制图形、处理用户输入等功能。你可以根据自己的需求进行进一步的定制和优化。希望这个示例能帮到你!
相关推荐
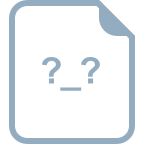
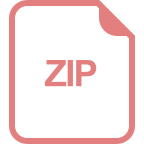
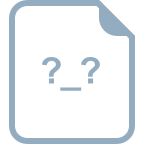














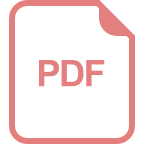