如何使用Python实现人脸识别技术并编写相应的代码?
时间: 2024-10-27 08:09:14 浏览: 42
在Python中实现人脸识别通常涉及到深度学习库,如OpenCV(用于图像处理)、Dlib(人脸检测)和Face_recognition(面部识别)。以下是简单的步骤和示例代码:
首先,你需要安装必要的库:
```bash
pip install opencv-python dlib face_recognition
```
然后,你可以通过以下步骤实现基础的人脸识别:
1. **人脸检测**:
使用Dlib库的`face_recognition_hog_face_detector`函数获取人脸位置:
```python
import cv2
from dlib import get_frontal_face_detector
detector = get_frontal_face_detector()
image = cv2.imread('your_image.jpg')
faces = detector(image)
```
2. **面部编码**:
对每个检测到的人脸,使用Face_recognition库的`face_encodings`方法计算特征向量:
```python
from face_recognition import face_encodings
known_faces = [] # 存储已知人脸的特征向量
known_names = [] # 对应的名字
for i, face in enumerate(faces):
encoding = face_encodings(image, [face])[0]
known_faces.append(encoding)
known_names.append('Person_' + str(i)) # 假设每个人有自己的名字
```
3. **人脸识别**:
将新的面部编码与已知的脸部进行比较,找到最相似的一个:
```python
def find_most_similar_face(face_to_compare, known_faces, known_names):
best_match_index = None
best_match_score = -1
for index, known_face in enumerate(known_faces):
similarity = compare_faces([face_to_compare], [known_face])
if similarity[0] > best_match_score:
best_match_index = index
best_match_score = similarity[0]
return best_match_index, known_names[best_match_index]
face_to_compare = face_encodings(image, [faces[0]])[0] # 测试人脸
match_index, matched_name = find_most_similar_face(face_to_compare, known_faces, known_names)
print("Detected face matches", matched_name)
```
4. **人脸比对**:
可能需要用到`compare_faces`函数来进行精确匹配。
注意,这只是一个基本的示例,并未涉及实际的情感分析、年龄估计等高级功能。此外,对于大规模的实时应用,你可能需要考虑性能优化和更复杂的框架,比如MTCNN或TensorFlow。
阅读全文
相关推荐
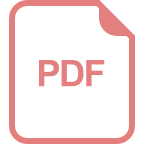
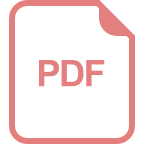
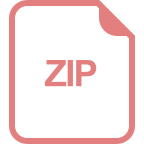
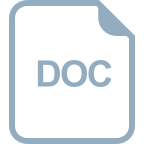
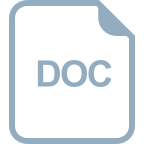
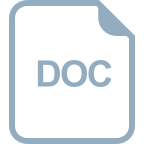
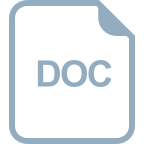
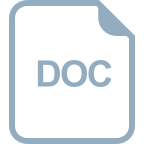



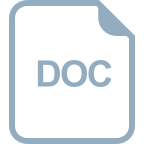
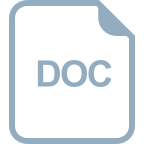
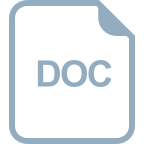
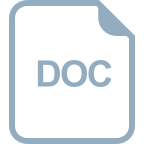
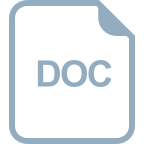
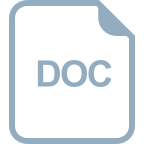

