Java解析Excel文件(.xlsx和.xls格式均适用)
时间: 2024-04-24 18:26:12 浏览: 178
你可以使用Apache POI库来解析Excel文件。下面是一个使用Java解析Excel文件的示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
public class ExcelParser {
public static void main(String[] args) {
String filePath = "path/to/your/excel/file.xlsx"; // 替换为你的Excel文件路径
try {
FileInputStream fis = new FileInputStream(new File(filePath));
Workbook workbook;
if (filePath.endsWith(".xlsx")) {
workbook = new XSSFWorkbook(fis); // 处理.xlsx文件
} else if (filePath.endsWith(".xls")) {
workbook = new HSSFWorkbook(fis); // 处理.xls文件
} else {
throw new IllegalArgumentException("The specified file is not Excel file");
}
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
for (Row row : sheet) {
for (Cell cell : row) {
CellType cellType = cell.getCellType();
if (cellType == CellType.STRING) {
System.out.print(cell.getStringCellValue() + " ");
} else if (cellType == CellType.NUMERIC) {
System.out.print(cell.getNumericCellValue() + " ");
} else if (cellType == CellType.BOOLEAN) {
System.out.print(cell.getBooleanCellValue() + " ");
}
}
System.out.println();
}
workbook.close();
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请将代码中的`"path/to/your/excel/file.xlsx"`替换为你实际的Excel文件路径。该代码会打开Excel文件并输出每个单元格的值。你可以根据需要对解析的内容进行进一步处理。
阅读全文
相关推荐
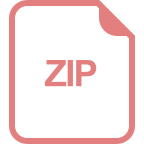
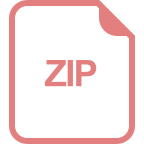
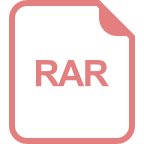
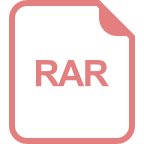
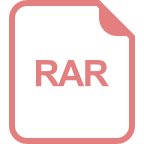
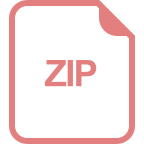
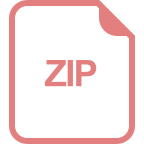
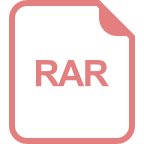
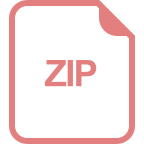
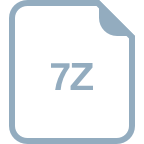
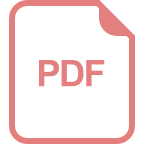
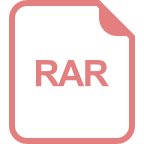
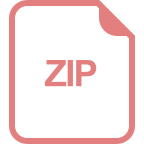
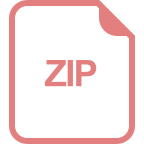
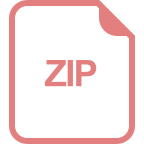
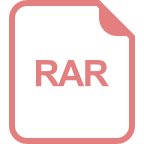
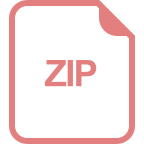
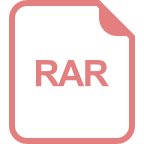