c获取鼠标点击的位置
时间: 2024-09-23 07:09:57 浏览: 39
在C语言中,直接获取鼠标点击位置通常不是标准库的一部分,因为C语言本身并不支持图形用户界面操作。如果你想在Windows平台上编写程序来获取鼠标位置,你需要利用Windows API函数,如`GetCursorPos()` 和 `SetCursorPos()`。
以下是一个简单的示例,展示了如何通过头文件`windows.h`来获取鼠标当前位置:
```c
#include <windows.h>
void get_mouse_pos(int* x, int* y) {
POINT pos;
GetCursorPos(&pos);
*x = pos.x;
*y = pos.y;
}
int main() {
int mouse_x, mouse_y;
get_mouse_pos(&mouse_x, &mouse_y);
printf("Mouse position: (%d, %d)\n", mouse_x, mouse_y);
return 0;
}
```
在这个例子中,`get_mouse_pos`函数接收两个整数指针,分别用于存储X坐标和Y坐标。注意这需要在Windows环境下运行,并且你可能需要链接到合适的库。
如果你在其他操作系统上,比如Linux或macOS,你可能需要用到不同的API,例如Xlib或Carbon APIs。
相关问题
motifi程序获取鼠标坐标位置程序
Motif是一个古老的图形用户界面工具包,在Unix-like操作系统上广泛使用,特别是在X Window System中。如果你想通过Motif编写一个程序来获取鼠标的位置,你可以使用MOTIF库中的` XmTranslateScreenEvent `函数。这个函数会将事件转换成屏幕坐标系的值。
以下是一个简单的例子:
```c
#include <Xm/Xm.h>
#include <stdio.h>
// 获取鼠标点击位置的函数
void getMousePosition(XEvent* event) {
int x = event->x_root;
int y = event->y_root;
printf("Mouse clicked at position (%d,%d)\n", x, y);
}
int main() {
Display *display = XOpenDisplay(NULL); // 打开显示连接
if (!display) {
perror("Could not open display");
return -1;
}
XtAppContext app_context = XtCreateApplicationContext(); // 创建应用上下文
XmMainWindow win, *appwin; // 主窗口指针
XtInitApplication(app_context);
win = XmCreateMainWindow(app_context, NULL, NULL, True, NULL);
appwin = (XmMainWindow *)XtParent(win);
// 注册鼠标按下事件处理器
XtAddEventHandler(appwin, XmNAnyButtonPressMask, True,
(XtPointer)&getMousePosition, Xt HobbitEventMask);
// 显示窗口并进入消息循环
XtRealizeWidget(win);
XtMainLoop();
XCloseDisplay(display);
return 0;
}
```
当你运行这个程序,当鼠标在窗口内被按下时,它就会打印出鼠标的当前位置。
c语言读取鼠标左键点击时刻的鼠标坐标
在C语言中,可以通过Windows API函数来读取鼠标左键点击时刻的鼠标坐标。具体步骤如下:
1. 在程序中包含Windows.h头文件。
2. 使用GetMessage函数获取消息。
3. 判断消息类型是否为鼠标左键按下消息(WM_LBUTTONDOWN)。
4. 获取鼠标坐标,将其存储到一个变量中。
以下是一个示例代码:
```
#include <Windows.h>
#include <stdio.h>
int main()
{
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
if (msg.message == WM_LBUTTONDOWN) // 判断消息类型
{
POINT p = { LOWORD(msg.lParam), HIWORD(msg.lParam) }; // 获取鼠标坐标
printf("Mouse left button down at (%d, %d)\n", p.x, p.y);
}
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
```
该代码会不断循环获取消息,并在收到鼠标左键按下消息时输出坐标。需要注意的是,该代码只能在Windows操作系统中运行。如果需要在其他操作系统中读取鼠标左键点击时刻的鼠标坐标,则需要使用该操作系统所支持的方法。
阅读全文
相关推荐
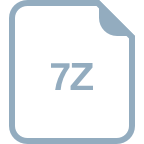
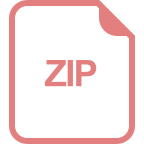
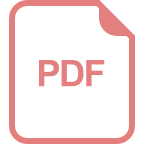
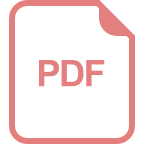
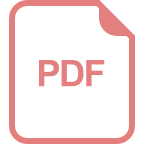
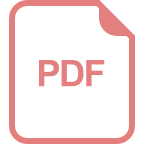
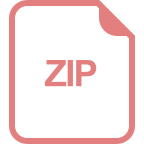
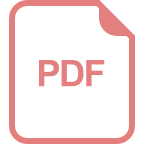
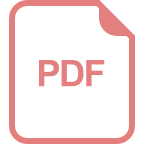
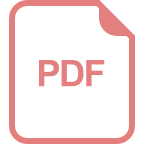
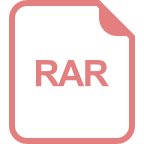
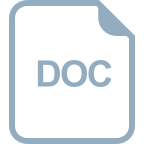


