qt同步异步串口通讯
时间: 2023-08-05 22:10:15 浏览: 263
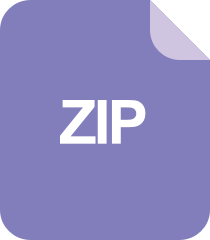
QT 串口通讯 头文件 动态库 静态库
在Qt中进行同步和异步串口通信,可以使用Qt的串口模块(QSerialPort)来实现。
对于同步串口通信,可以使用阻塞的方式进行。以下是一个简单的同步串口通信的示例:
```cpp
#include <QCoreApplication>
#include <QSerialPort>
#include <QSerialPortInfo>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 查找可用的串口
QList<QSerialPortInfo> portList = QSerialPortInfo::availablePorts();
if (portList.isEmpty()) {
qDebug() << "No serial ports found.";
return 0;
}
// 打开串口
QSerialPort serial;
serial.setPort(portList.first());
if (!serial.open(QIODevice::ReadWrite)) {
qDebug() << "Failed to open serial port.";
return 0;
}
// 设置串口参数
serial.setBaudRate(QSerialPort::Baud9600);
serial.setDataBits(QSerialPort::Data8);
serial.setParity(QSerialPort::NoParity);
serial.setStopBits(QSerialPort::OneStop);
serial.setFlowControl(QSerialPort::NoFlowControl);
// 发送数据
QByteArray sendData = "Hello, serial port!";
serial.write(sendData);
// 等待接收数据
if (serial.waitForReadyRead(3000)) {
QByteArray receivedData = serial.readAll();
qDebug() << "Received data: " << receivedData;
} else {
qDebug() << "Timeout occurred while waiting for data.";
}
// 关闭串口
serial.close();
return a.exec();
}
```
对于异步串口通信,可以使用Qt的信号槽机制来处理串口事件。以下是一个简单的异步串口通信的示例:
```cpp
#include <QCoreApplication>
#include <QSerialPort>
#include <QSerialPortInfo>
#include <QDebug>
class SerialPortHandler : public QObject
{
Q_OBJECT
public:
SerialPortHandler(QObject *parent = nullptr) : QObject(parent)
{
// 查找可用的串口
QList<QSerialPortInfo> portList = QSerialPortInfo::availablePorts();
if (portList.isEmpty()) {
qDebug() << "No serial ports found.";
return;
}
// 打开串口
serial.setPort(portList.first());
if (!serial.open(QIODevice::ReadWrite)) {
qDebug() << "Failed to open serial port.";
return;
}
// 设置串口参数
serial.setBaudRate(QSerialPort::Baud9600);
serial.setDataBits(QSerialPort::Data8);
serial.setParity(QSerialPort::NoParity);
serial.setStopBits(QSerialPort::OneStop);
serial.setFlowControl(QSerialPort::NoFlowControl);
// 连接串口的readyRead信号到槽函数
connect(&serial, &QSerialPort::readyRead, this, &SerialPortHandler::handleReadyRead);
}
~SerialPortHandler()
{
// 关闭串口
serial.close();
}
public slots:
void handleReadyRead()
{
// 接收数据
QByteArray receivedData = serial.readAll();
qDebug() << "Received data: " << receivedData;
}
private:
QSerialPort serial;
};
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
SerialPortHandler handler;
return a.exec();
}
#include "main.moc"
```
在这个例子中,我们创建了一个名为SerialPortHandler的类,它继承自QObject,并处理串口的readyRead信号。在主函数中,我们创建了一个SerialPortHandler对象,从而启动了异步串口通信。
这只是Qt串口通信的基本示例,你可以根据自己的需求进行进一步的扩展和调整。希望对你有帮助!
阅读全文
相关推荐
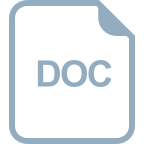
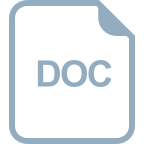
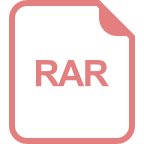
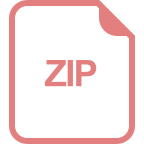
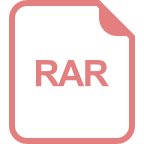
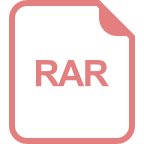
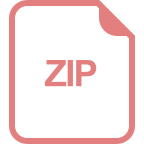
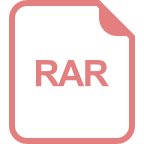
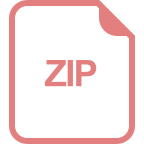
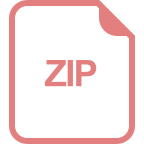
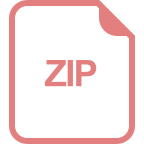
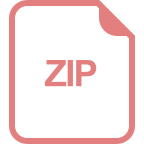
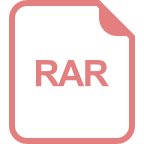
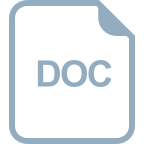

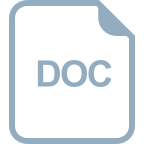
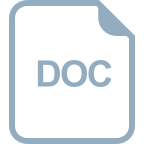
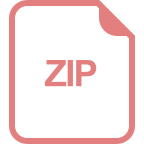