使用Python的request,bs4,jieba,wordcloud,matplotlib模块编写一个爬虫,爬取学术新闻信息,进行分词,实现可视化,并绘制出词出现的条形统计图
时间: 2024-12-23 10:20:03 浏览: 2
好的,首先我们需要安装必要的库,如`requests`, `beautifulsoup4`, `jieba`, `wordcloud` 和 `matplotlib`。然后我们可以按照以下步骤创建一个简单的学术新闻信息爬虫、分词和可视化流程:
1. **导入所需库**:
```python
import requests
from bs4 import BeautifulSoup
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
```
2. **获取网页内容**:
```python
def get_news(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
print(f"Failed to fetch URL: {response.status_code}")
return None
url = "https://example.com/academic_news" # 替换为你想要爬取的学术新闻页面
news_text = get_news(url)
if news_text is not None:
soup = BeautifulSoup(news_text, 'html.parser')
```
3. **提取文章文本并分词**:
```python
# 提取文章正文
content = soup.find('div', class_='article-content') or soup.find('p')
text = content.get_text().replace('\n', '')
# 分词
words = jieba.lcut(text)
```
4. **生成词云**:
```python
wordcloud = WordCloud(font_path='simhei.ttf', width=800, height=600).generate_from_frequencies(dict(jieba.Counter(words)))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
5. **统计词频并制作条形图**:
由于WordCloud并不直接支持生成条形图,我们先需要将分词结果转换成频率字典,然后用`collections.Counter`计算词频,最后通过`matplotlib`画图:
```python
from collections import Counter
word_freq = dict(Counter(words))
sorted_word_freq = sorted(word_freq.items(), key=lambda x: x[1], reverse=True)
plt.barh(range(len(sorted_word_freq)), [freq for freq, _ in sorted_word_freq])
plt.yticks(range(len(sorted_word_freq)), [word for _, word in sorted_word_freq])
plt.xlabel("词频")
plt.title("学术新闻关键词条形图")
plt.show()
```
阅读全文
相关推荐
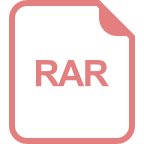
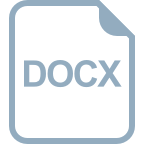
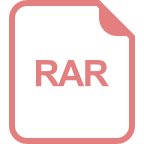
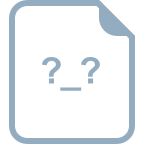
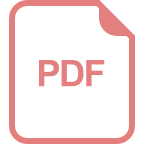
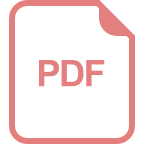
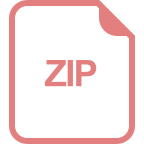
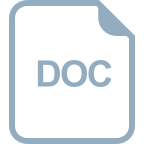
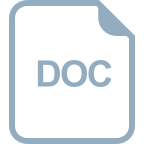
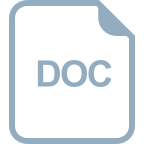







