vue require动态路由打包
时间: 2023-08-19 07:06:10 浏览: 179
Vue.js 中的动态路由可以通过 `require` 函数来实现按需加载和打包。
首先,你需要在路由配置中使用 `component: () => require('your-component-path')` 来定义动态路由。这里的 `'your-component-path'` 是你要加载的组件的路径。
接下来,当该路由被访问时,Vue.js 会动态加载该组件。使用 `require` 函数可以将组件分割成更小的代码块,并在需要的时候进行按需加载,从而减小初始加载的文件大小。
在打包时,Webpack 会根据路由配置自动将这些动态加载的组件进行代码拆分和打包。这样,每个动态路由都会生成一个单独的打包文件,当该路由被访问时才会加载对应的文件。
总结起来,动态路由的打包可以通过在路由配置中使用 `require` 函数来实现按需加载和打包。这样可以提高应用的性能和加载速度。
相关问题
electron 打包vue 路由无法跳转
这个问题可能与你的路由配置有关。在使用 Electron 打包 Vue 时,需要注意以下几点:
1. 确保你的路由配置正确。在开发过程中可能会使用 `history` 模式,而在 Electron 中需要使用 `hash` 模式。你需要将路由模式修改为 `hash` 模式,可以在 `router/index.js` 中添加如下代码:
```
const router = new VueRouter({
mode: 'hash',
routes: [...]
})
```
2. 确保你的页面正常加载。在 Electron 中,你需要使用绝对路径来引用你的页面。在你的路由配置中,确保你的组件路径使用绝对路径,例如:
```
{
path: '/about',
component: require('@/views/About.vue').default
}
```
3. 确保你的页面可以正常加载外部资源。在 Electron 中,你的页面不再受同源策略的限制,因此你可以在页面中加载外部资源。但是,你需要在你的 `main.js` 文件中添加如下代码来禁用安全策略:
```
const { app, BrowserWindow } = require('electron')
app.commandLine.appendSwitch('disable-web-security')
app.commandLine.appendSwitch('allow-file-access-from-files')
```
以上是一些常见的解决方法,如果以上方法都无法解决你的问题,你可以提供更多的信息,例如你的代码、错误信息等。
vue2打包 按需加载路由
Vue2的按需加载路由主要是为了提高应用的初始加载速度和性能,特别是在大型项目中有大量的路由的情况下。Webpack是一个常用的前端构建工具,它允许我们配置按需加载。在Vue Router中,可以结合Webpack的Code Splitting功能实现按需加载。
以下是一般的步骤:
1. **安装依赖**:首先需要安装`vue-router`和相关的插件,如`@vue/cli-plugin-router`如果使用Vue CLI。
```bash
npm install vue-router webpack-bundle-analyzer --save-dev
```
2. **配置`webpack.config.js`**:在`configureWebpack`选项中添加按需加载的配置。例如,使用动态导入(import())和 HtmlWebpackPlugin来分割模块:
```javascript
module.exports = {
//...
configureWebpack: {
optimization: {
splitChunks: {
chunks: 'all',
name: 'chunk-vendors', // 子模块名
minSize: 0,
maxSize: 0,
cacheGroups: {
vendors: { test: /[\\/]node_modules[\\/]/, priority: -10, chunks: 'initial' },
common: { test: /[\\/]common[\\/].*\.js/, priority: -20, reuseExistingChunk: true, chunks: 'all' }
}
}
},
runtimeChunk: {
name: 'runtime'
}
}
};
```
3. **设置路由**:在`router/index.js`中,使用异步组件来声明按需加载的路由:
```javascript
const AsyncComponent = function (resolve) {
require(['path/to/your/module'], resolve);
};
export default [
{ path: '*', component: AsyncComponent }
];
```
4. **在模板中引用**:在需要的地方,通过`<router-view>`渲染异步组件:
```html
<template>
<div>
<router-view :component="loadComponent"></router-view>
</div>
</template>
<script>
import AsyncComponent from '@/components/YourComponent.vue';
export default {
data() {
return {
loadComponent: () => AsyncComponent
}
}
}
</script>
```
阅读全文
相关推荐
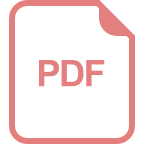
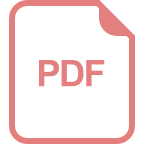
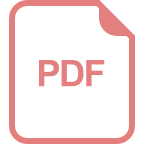













