selenium点击按键
时间: 2023-09-12 12:13:32 浏览: 125
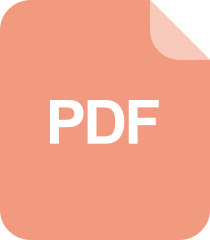
selenium webdriver

要在Selenium中模拟点击按键,您可以使用`Keys`类提供的方法。以下是一个示例,显示如何点击回车键:
```python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# 初始化webdriver
driver = webdriver.Chrome()
# 打开网页
driver.get("https://www.example.com")
# 定位到输入框元素
input_element = driver.find_element_by_id("input-box")
# 输入内容并按下回车键
input_element.send_keys("Hello, World!")
input_element.send_keys(Keys.RETURN)
```
这个例子中,我们使用了Chrome浏览器并找到了一个id为"input-box"的输入框元素。然后,我们使用`send_keys()`方法输入文本并通过`Keys.RETURN`模拟按下回车键。
您可以根据自己的需求修改代码来适应不同的场景和按键操作。
阅读全文
相关推荐
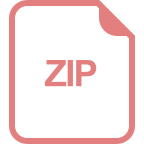
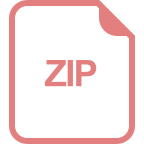
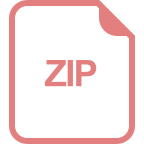
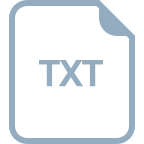
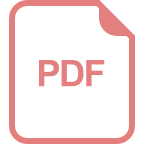
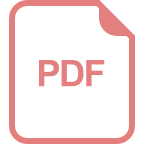
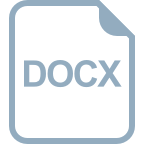
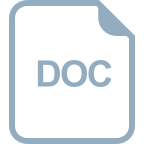







