基于OpenGl的图形的缩放,旋转的平移完整代码
时间: 2024-09-27 09:10:39 浏览: 6
OpenGL是一种用于渲染2D和3D图形的强大API,它的变换操作主要包括缩放、旋转和平移。以下是基于OpenGL ES(针对移动设备的简化版)的一个简单示例,展示了如何分别对模型进行这些变换:
```cpp
// 引入必要的头文件
#include <EGL/egl.h>
#include <GLES2/gl2.h>
// 缩放矩阵函数
void scale(float scale_factor) {
GLfloat matrix[16] = {1, 0, 0, 0,
0, 1, 0, 0,
0, 0, scale_factor, 0,
0, 0, 0, 1};
glMultMatrixf(matrix);
}
// 旋转矩阵函数
void rotate(float angle, float x, float y, float z) {
const float cosVal = cos(angle);
const float sinVal = sin(angle);
GLfloat matrix[16] = {
cosVal * x*x + sinVal * z*z, cosVal * x*y - sinVal * z * x, cosVal * x*z + sinVal * y * z, 0,
cosVal * x*y + sinVal * z * x, cosVal * y*y + sinVal * z * z, cosVal * y * z - sinVal * x * z, 0,
cosVal * x*z - sinVal * y * z, cosVal * y * z + sinVal * x * z, cosVal * z * z + sinVal * x * x, 0,
0, 0, 0, 1
};
glMultMatrixf(matrix);
}
// 平移矩阵函数
void translate(float x, float y, float z) {
GLfloat matrix[16] = {1, 0, 0, x,
0, 1, 0, y,
0, 0, 1, z,
0, 0, 0, 1};
glMultMatrixf(matrix);
}
// 在绘制模型之前应用变换
void applyTransformations(float scale, float rotation_angle, float translation_x, float translation_y, float translation_z) {
scale(scale);
rotate(rotation_angle, 1.0, 0.0, 0.0); // 通常将x轴作为旋转轴
translate(translation_x, translation_y, translation_z);
}
// ... 在你的主循环中,在绘制之前调用applyTransformations()
```
注意这只是一个基本的示例,实际使用时可能会需要处理更复杂的变换组合,并考虑模型空间和视图空间的转换。同时,记得在每次变换后调用`glPopMatrix()`以恢复到上一层的变换状态。
相关推荐
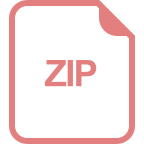
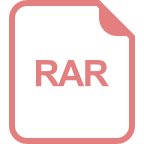
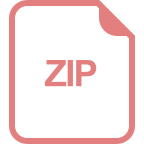
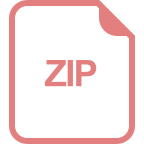
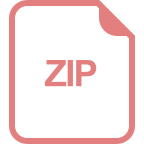
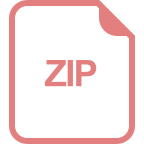
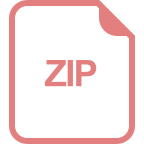
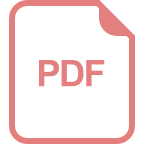
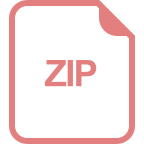
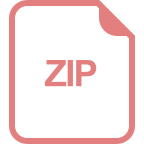
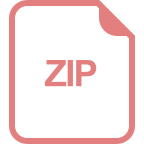
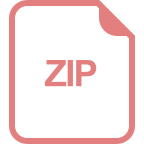