在这样结构下动态加载 css 文件 import { onMounted, onUnmounted } from "vue"; const props = defineProps<{ components: any[]; style?: string; }>(); onMounted(() => { if (props.style) { console.log("mounted: " + props.style); } }); onUnmounted(() => { if (props.style) { console.log("unmounted: " + props.style); } });
时间: 2024-04-18 14:30:32 浏览: 197
在你提供的代码中,使用了 Vue 3 的 Composition API 中的 `onMounted` 和 `onUnmounted` 钩子函数。根据代码逻辑,当组件被挂载或卸载时,会执行对应的回调函数。
在 `onMounted` 钩子函数中,你检查了 `props.style` 的值是否存在,如果存在,则输出相应的信息到控制台。
在 `onUnmounted` 钩子函数中,同样检查了 `props.style` 的值是否存在,如果存在,则输出相应的信息到控制台。
这段代码的作用是在组件挂载和卸载时,根据传入的 `style` 属性值进行相关操作。如果传入 `style` 属性,则在组件挂载时输出 `"mounted: " + props.style` 到控制台,而在组件卸载时输出 `"unmounted: " + props.style` 到控制台。
需要注意的是,这段代码中并没有动态加载 CSS 文件的逻辑,只是通过控制台输出了一些信息。如果你希望动态加载 CSS 文件,你需要使用其他方法,比如创建 `<link>` 元素并添加到文档中。
相关问题
这个有问题吗?<!-- src/components/layout/DefaultLayout.vue --> <script setup lang="ts"> import { onMounted, onUnmounted, ref } from "vue"; const props = defineProps<{ components: any[]; style?: string; }>(); const link = ref<HTMLLinkElement | null>(); onMounted(() => { if (props.style && props.style.endsWith(".css")) { const element = document.createElement("link"); element.rel = "stylesheet"; element.rel = props.style; element.type = "text/css"; link.value = element; } }); onUnmounted(() => { if (link.value) { link.value.remove(); link.value = null; } }); </script> <template> <component v-for="item in components" :is="item.component" :class="item.style" :="item.props" @="item.emits" /> </template> <style scoped></style>
根据你提供的代码片段,在 `DefaultLayout.vue` 组件中使用了 `<script setup>` 的方式来编写脚本,这是 Vue 3 中的新特性之一。代码片段中使用了 `onMounted` 和 `onUnmounted` 钩子函数,并创建了一个名为 `link` 的 `ref` 引用。
在 `onMounted` 钩子函数中,通过判断 `props.style` 是否存在,并且以 `.css` 结尾,来创建 `<link>` 元素,并设置相关属性。然后将创建的元素赋值给 `link` 引用。
在 `onUnmounted` 钩子函数中,检查 `link` 引用是否存在,如果存在,则移除元素并将 `link` 引用置为 `null`。
在模板中使用了 `v-for` 循环渲染 `components` 数组中的组件,并传递了相应的属性和事件。
至于你提到的问题,从这个代码片段来看,没有明显的问题。但是请确保在使用 `<script setup>` 时已正确导入相关的函数和变量,例如 `defineProps` 和 `ref`。
另外,需要注意的是,在模板中的动态绑定属性部分,你的代码片段中的语法似乎存在一些问题。请确保这部分的语法是正确的。
如果还有其他问题或者提供更多上下文信息,请随时告诉我。
这样会报 v-on with no argument expects an object value. <script setup lang="ts"> import { onMounted, onUnmounted } from "vue"; const props = defineProps<{ components: any[]; style?: string; }>(); let linkElement: HTMLLinkElement | null = null; onMounted(() => { if ( props.style && (props.style.endsWith(".css") || props.style.startsWith("http")) ) { linkElement = document.createElement("link"); linkElement.rel = "stylesheet"; linkElement.href = props.style; linkElement.type = "text/css"; document.head.appendChild(linkElement); } }); onUnmounted(() => { if (linkElement) { document.head.removeChild(linkElement); linkElement = null; } }); </script> <template> <main> <component v-for="item in components" :is="item.component" :class="item.style" :="item.props" @="item.emits" /> </main> </template>
根据你提供的代码,出现警告的原因是在模板中的 `@` 符号处,没有提供具体的事件名称。在模板中使用 `v-on` 指令时,需要提供一个对象来指定事件名和对应的事件处理函数。
要解决这个问题,你需要为 `v-on` 指令传递一个事件对象,其中键是事件名,值是对应的事件处理函数。例如,你可以修改模板代码如下:
```vue
<template>
<main>
<component
v-for="item in components"
:is="item.component"
:class="item.style"
:="item.props"
v-on="item.emits"
/>
</main>
</template>
```
在这里,我将 `@` 符号替换为了 `v-on`,并将 `item.emits` 作为一个对象传递给了 `v-on` 指令。
请确保在 `item.emits` 中提供正确的事件名称和对应的事件处理函数。
如果还有其他问题,请随时提问。
阅读全文
相关推荐
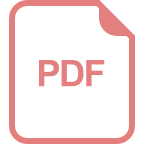
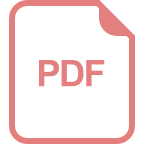
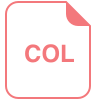
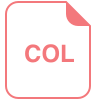
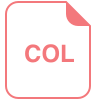
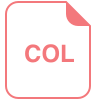
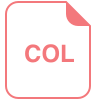




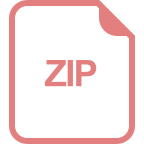
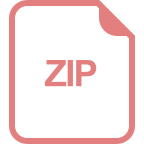
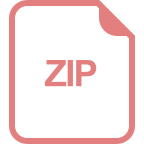
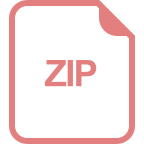