C++ 清理std::vector<std::pai<std::string,std::string>>类型中的相同键的值
时间: 2024-02-23 10:54:26 浏览: 189
要清理std::vector<std::pair<std::string, std::string>>类型中的相同键的值,可以按照以下步骤进行操作:
1. 首先,创建一个空的std::map<std::string, std::string>,用于存储唯一的键值对。
2. 遍历std::vector中的每个元素。
3. 对于每个元素,检查其键是否已经存在于std::map中。
4. 如果键不存在于std::map中,将该键值对插入到std::map中。
5. 如果键已经存在于std::map中,则根据需求进行处理,比如覆盖旧值或者忽略新值。
6. 最后,将std::map中的键值对重新存储回std::vector中。
下面是示例代码:
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <utility>
int main() {
std::vector<std::pair<std::string, std::string>> vec = {{"key1", "value1"}, {"key2", "value2"}, {"key1", "value3"}};
std::map<std::string, std::string> uniqueMap;
for (const auto& pair : vec) {
const auto& key = pair.first;
const auto& value = pair.second;
// 检查键是否已经存在于std::map中
if (uniqueMap.find(key) == uniqueMap.end()) {
// 键不存在,插入到std::map中
uniqueMap[key] = value;
} else {
// 键已经存在,根据需求进行处理,比如覆盖旧值或者忽略新值
// 这里示例为覆盖旧值
uniqueMap[key] = value;
}
}
// 清空原始的std::vector
vec.clear();
// 将std::map中的键值对重新存储回std::vector中
for (const auto& pair : uniqueMap) {
vec.push_back(pair);
}
// 输出结果
for (const auto& pair : vec) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
这段代码会输出去重后的键值对。你可以根据实际需求修改处理相同键的方式。
阅读全文
相关推荐
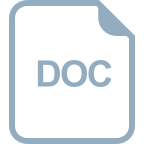
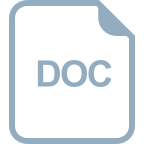
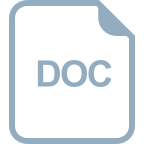


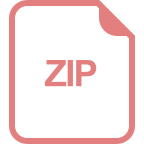
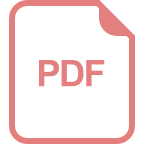
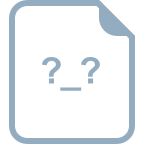
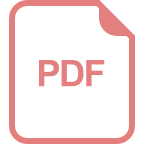
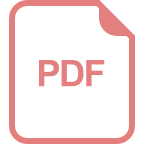
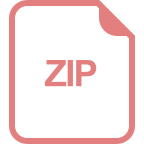
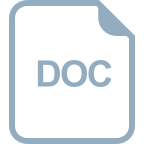
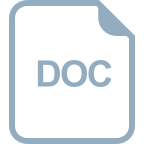
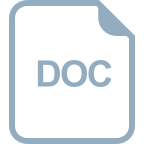

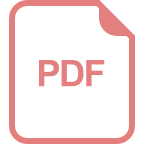
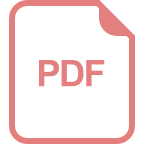
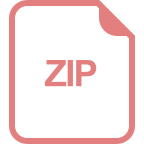
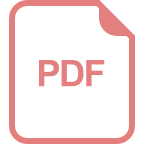