设计一个c++算法统计在输入字符串中各个不同字符出现的频度并将结果存入文件。
时间: 2024-12-23 15:15:36 浏览: 9
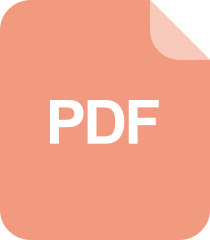
C++ 读文件 将文件内容读入到字符串string中的方法
为了设计一个C++算法统计输入字符串中各个不同字符的频度并存储到文件中,我们可以按照以下步骤进行:
1. 创建一个`std::unordered_map`,键(key)为字符,值(value)为该字符的出现次数。
2. 遍历输入字符串中的每一个字符,检查`std::unordered_map`中是否已经存在该字符。若不存在,初始化其计数为1;已存在则递增计数。
3. 当遍历结束后,打开一个文件流,例如`ofstream`,准备将统计信息写入文件。
4. 使用循环遍历`std::unordered_map`,对于每个字符及其出现次数,写入文件。
5. 关闭文件流,结束程序。
以下是示例代码实现:
```cpp
#include <iostream>
#include <fstream>
#include <unordered_map>
void countCharacters(const std::string& input_string, const std::string& output_file) {
std::unordered_map<char, int> char_counts;
for (char c : input_string) {
if (isalpha(c)) { // 只统计字母字符,忽略非字母
char_counts[c]++;
}
}
std::ofstream outfile(output_file);
if (!outfile.is_open()) {
std::cerr << "Failed to open file." << std::endl;
return;
}
for (const auto& pair : char_counts) {
outfile << pair.first << ": " << pair.second << "\n";
}
outfile.close();
}
int main() {
std::string input_str = "Hello, World!";
std::string output_path = "character_counts.txt";
countCharacters(input_str, output_path);
return 0;
}
```
运行此程序后,会创建一个名为`character_counts.txt`的文件,内容类似:"H: 1", "e: 1", "l: 3", "o: 2", "W: 1", "r": 1, "d: 1"。
阅读全文
相关推荐
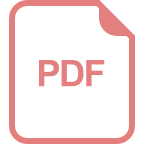
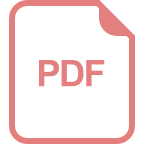
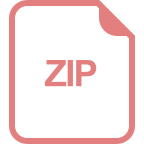
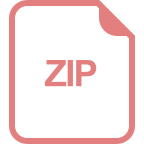
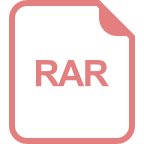
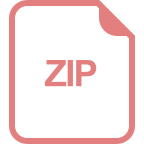
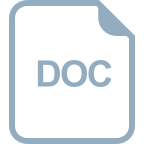
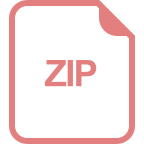
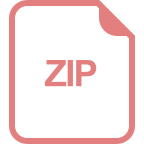
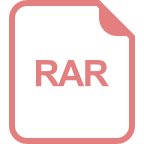
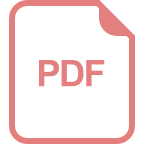
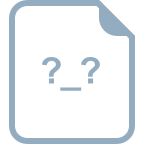
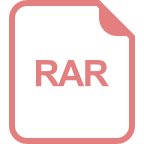
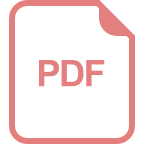
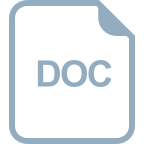
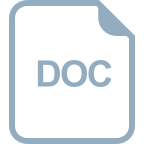