6、输入一个字符串,如果字符串是一个回文字符串,返回 true;否则,返回 false。 回文数 是指正序(从左向右)和倒序(从右向左)读都是一样的字符串。 例如,aca 是回文,而 abc 不是。 c语言
时间: 2024-10-14 12:06:33 浏览: 33
在 C 语言中,判断一个字符串是否是回文可以采用双指针法。通常的做法是从字符串两端开始比较字符,同时向中间移动,直到两个指针相遇或交叉。如果在这个过程中,任意一对对应位置的字符不相等,则该字符串不是回文;如果所有字符都匹配,则它是回文。
下面是一个简单的 C 程序示例,用于检查给定的字符串是否为回文:
```c
#include <stdbool.h>
#include <stdio.h>
#include <string.h>
bool isPalindrome(char str[]) {
int start = 0;
int end = strlen(str) - 1; // 获取字符串长度
while (start < end) {
if (str[start] != str[end]) {
return false; // 如果不相等,立即返回false
}
start++; // 左指针向右移动
end--; // 右指针向左移动
}
return true; // 所有字符都匹配,返回true
}
int main() {
char input[100];
printf("Enter a string: ");
fgets(input, sizeof(input), stdin);
input[strcspn(input, "\n")] = '\0'; // 清除fgets产生的换行符
if (isPalindrome(input)) {
printf("%s is a palindrome.\n", input);
} else {
printf("%s is not a palindrome.\n", input);
}
return 0;
}
```
阅读全文
相关推荐
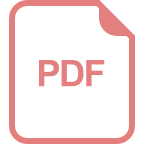
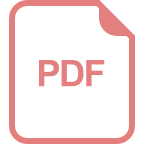
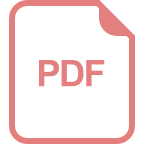













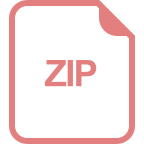
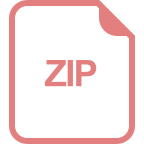