解析一下这行代码Set<String> groupSet = listMap.keySet();
时间: 2024-04-24 07:22:17 浏览: 10
这行代码的作用是将 `listMap` 中所有的键(key)存储到一个 Set 集合中,这个 Set 集合中的元素都是 String 类型的。可以通过这个 Set 集合来获取 `listMap` 中所有的键。
具体来说,`listMap` 是一个 Map 集合,它存储了一些键值对(key-value pair)。`keySet()` 方法是 Map 接口中的一个方法,用于获取 Map 中所有的键,返回一个 Set 集合。这个方法返回的 Set 集合中的元素是无序的,并且不允许有重复元素。这个 Set 集合可以用于迭代 Map 中的所有键,或者用于判断某个键是否存在于 Map 中。
将 `listMap.keySet()` 的返回值赋值给 `groupSet`,就创建了一个新的 Set 集合,其中包含了 `listMap` 中所有的键。这个 Set 集合的泛型参数是 `String`,这意味着集合中的元素都是 String 类型。
相关问题
Set<String> StringSet1 = Global.followMap.keySet();改为python
在 Python 中,可以使用以下代码创建一个 set 类型的变量 StringSet1,并将其赋值为 Global.followMap 中所有 key 的集合:
```
StringSet1 = set(Global.followMap.keys())
```
其中,set() 函数用于创建一个空的 set 集合,Global.followMap.keys() 返回一个包含 Global.followMap 中所有 key 的集合,使用 set() 函数将其转换为 set 集合后赋值给变量 StringSet1。
需要注意的是,在 Python 中,set 类型的变量没有 key 和 value 的区分,只有元素的集合。因此,如果需要获取 set 变量中的元素,可以使用 for 循环遍历,例如:
```
for item in StringSet1:
print(item)
```
上述代码将遍历 StringSet1 中的所有元素,并将其打印到控制台上。
Set<String> keySets=map.keySet();
这行代码将返回一个 Set 对象,其中包含了 HashMap 中所有键的集合。在这个例子中,我们将这个集合赋值给名为 keySets 的变量。通过调用 HashMap 的 `keySet()` 方法,我们可以获取 HashMap 中所有键的集合,并将其存储在一个 Set 对象中。这样,我们就可以通过遍历这个 Set 对象来访问 HashMap 中的所有键。
相关推荐
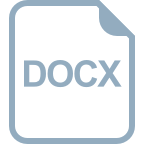
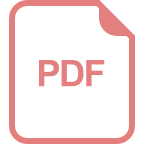












