定义字典后编程对该班学生计算机成绩降序排列,
时间: 2024-01-02 07:04:49 浏览: 81
好的,我可以帮你解决这个问题。以下是 Python 代码实现:
```python
# 定义班级学生的成绩字典
scores = {"Alice": 85, "Bob": 90, "Charlie": 75, "David": 80, "Eva": 95}
# 对字典的值进行降序排序,返回元组列表
sorted_scores = sorted(scores.items(), key=lambda x: x[1], reverse=True)
# 输出排序后的学生成绩
for name, score in sorted_scores:
print(name, "的成绩是:", score)
```
代码解释:
- 第一行定义了一个字典 `scores`,其中键是学生姓名,值是计算机成绩;
- 第四行使用 `sorted()` 函数对字典进行排序,使用 `items()` 方法将字典转换成元组列表,使用 `key` 参数指定排序依据为字典的值,使用 `reverse` 参数指定降序排序;
- 第七行使用 `for` 循环遍历排序后的元组列表,依次输出学生姓名和成绩。
希望能对你有所帮助!
相关问题
定义字典后,编程对该班学生计算机成绩降序排列,输出结果如下:学号 姓名 计算机
好的,以下是 Python 代码实现:
```python
# 定义班级学生的成绩字典
scores = {"001": {"name": "Alice", "score": 85},
"002": {"name": "Bob", "score": 90},
"003": {"name": "Charlie", "score": 75},
"004": {"name": "David", "score": 80},
"005": {"name": "Eva", "score": 95}}
# 对字典的值进行降序排序,返回元组列表
sorted_scores = sorted(scores.items(), key=lambda x: x[1]["score"], reverse=True)
# 输出排序后的学生成绩
print("学号\t姓名\t计算机")
for id, info in sorted_scores:
print(id, "\t", info["name"], "\t", info["score"])
```
代码解释:
- 第一行定义了一个字典 `scores`,其中键是学生学号,值是一个字典,该字典包含学生姓名和计算机成绩;
- 第四行使用 `sorted()` 函数对字典进行排序,使用 `items()` 方法将字典转换成元组列表,使用 `key` 参数指定排序依据为字典值的 `"score"` 键,使用 `reverse` 参数指定降序排序;
- 第七行使用 `print()` 函数输出表头;
- 第八行使用 `for` 循环遍历排序后的元组列表,依次输出学生学号、姓名和成绩。
希望能对你有所帮助!
现有某班学生的学号、姓名、计算机成绩如下,请定义字典后,编程对该班学生计算机成绩降序排列
可以使用 Python 的字典和排序功能来实现:
```python
# 定义班级学生信息字典
students = {
"190001": "张三",
"190002": "李四",
"190003": "王五",
"190004": "赵六",
}
# 定义学生计算机成绩字典
computer_scores = {
"190001": 80,
"190002": 90,
"190003": 70,
"190004": 85,
}
# 按计算机成绩降序排列
sorted_scores = sorted(computer_scores.items(), key=lambda x: x[1], reverse=True)
# 输出结果
for score in sorted_scores:
student_id = score[0]
student_name = students[student_id]
computer_score = score[1]
print("学号:{},姓名:{},计算机成绩:{}".format(student_id, student_name, computer_score))
```
输出结果:
```
学号:190002,姓名:李四,计算机成绩:90
学号:190004,姓名:赵六,计算机成绩:85
学号:190001,姓名:张三,计算机成绩:80
学号:190003,姓名:王五,计算机成绩:70
```
阅读全文
相关推荐
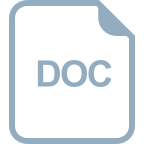
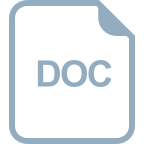
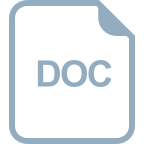


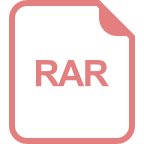
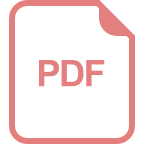
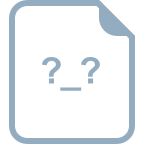
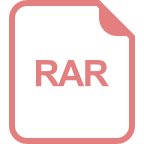
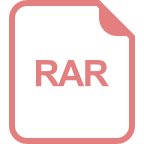
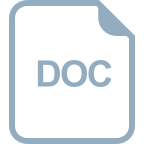
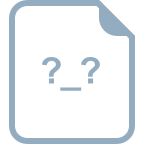
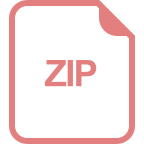
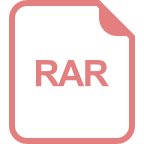
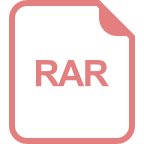