用 Java netty写即时通讯软件
时间: 2023-05-29 12:02:41 浏览: 58
作为AI语言模型,我能帮助您了解Java Netty和即时通讯软件的一些基本概念和步骤。下面是可能的大致步骤:
1. 确定通讯协议:在选择使用 Java Netty 开发即时通讯软件时,您需要确定使用的通信协议,如 TCP 或 UDP 协议,以便确保通信的可靠性和效率。
2. 编写服务器端代码:编写服务器端代码时,需要使用 Java Netty 框架来实现服务器功能。您需要定义协议和数据结构,处理连接、传输和接收数据等。
3. 编写客户端代码:编写客户端代码时,需要使用 Java Netty 框架来实现客户端功能。您需要定义协议和数据结构、处理连接、传输和接收数据。
4. 测试通讯功能:完成服务器和客户端的开发后,需要通过一些基本测试来确保通讯功能正常工作。
5. 实现更高级的功能:如果需要更高级的即时通讯功能,例如群聊、语音、视频通话等,您需要通过扩展协议和数据结构、调整服务器和客户端代码来实现这些功能。需要根据需求实现相应的数据结构、编码和解码逻辑。
6. 部署软件:将服务器端代码放在云服务上或自己的服务器上,或将客户端软件发布到应用商城或网站上,供用户下载和使用。
希望这些大致步骤能帮助您了解 Java Netty 编写即时通讯软件的一些基本流程和应该需要的步骤。但具体实现需遵守相关技术规范和安全防范措施,请根据实际情况和自己的技术储备进行具体实施。
相关问题
用java写一个netty的即时通讯demo
抱歉,作为AI语言模型,我无法编写实际的代码,但是我可以向您提供一些基本的步骤和代码示例,以便您开始编写Netty即时通信Demo。
1. 导入Netty依赖
在您的Maven项目中,将以下依赖项添加到pom.xml文件中:
```
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.65.Final</version>
</dependency>
```
2. 创建服务器
创建一个Netty服务器来接收客户端连接并处理消息:
```
public class ChatServer {
private final int port;
public ChatServer(int port) {
this.port = port;
}
public void start() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ChatServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
```
3. 创建消息处理器
创建一个消息处理器来处理客户端发送的消息:
```
public class ChatServerHandler extends SimpleChannelInboundHandler<String> {
private static final Map<Channel, String> channelMap = new ConcurrentHashMap<>();
private static final String CHAT_SERVER = "ChatServer";
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
Channel channel = ctx.channel();
String remoteAddress = channel.remoteAddress().toString();
channelMap.put(channel, remoteAddress);
System.out.println("Client " + remoteAddress + " connected to " + CHAT_SERVER);
super.channelActive(ctx);
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
Channel channel = ctx.channel();
String remoteAddress = channel.remoteAddress().toString();
channelMap.remove(channel);
System.out.println("Client " + remoteAddress + " disconnected from " + CHAT_SERVER);
super.channelInactive(ctx);
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, String message) throws Exception {
Channel channel = ctx.channel();
String remoteAddress = channel.remoteAddress().toString();
System.out.println("Received message from " + remoteAddress + ": " + message);
for (Channel ch : channelMap.keySet()) {
if (ch != channel) {
ch.writeAndFlush("[" + remoteAddress + "]: " + message + "\n");
}
}
}
}
```
4. 创建客户端
创建一个Netty客户端来连接服务器并发送消息:
```
public class ChatClient {
private final String host;
private final int port;
public ChatClient(String host, int port) {
this.host = host;
this.port = port;
}
public void start() throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ChatClientHandler());
}
});
ChannelFuture f = b.connect(host, port).sync();
BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
while (true) {
String message = in.readLine();
if (message == null) {
break;
}
f.channel().writeAndFlush(message + "\n");
}
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
}
```
5. 创建客户端消息处理器
创建一个消息处理器来处理从服务器接收的消息:
```
public class ChatClientHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String message) throws Exception {
System.out.println(message);
}
}
```
6. 运行Demo
在主方法中创建服务器和客户端实例并运行它们:
```
public class ChatDemo {
private static final int PORT = 8080;
private static final String HOST = "localhost";
public static void main(String[] args) throws Exception {
ChatServer server = new ChatServer(PORT);
ChatClient client = new ChatClient(HOST, PORT);
new Thread(() -> {
try {
server.start();
} catch (Exception e) {
e.printStackTrace();
}
}).start();
client.start();
}
}
```
现在您已经有了一个简单的Netty即时通信Demo,可以在本地计算机上启动服务器和客户端,并通过控制台输入消息进行通信。
netty实现即时通讯
Netty是一款基于NIO的网络通讯框架,它提供了高性能、高可靠性的网络编程能力,因此可以用来实现即时通讯。以下是一个简单的使用Netty实现即时通讯的示例:
1. 创建一个Netty的Server端和Client端
```java
// Server端
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new ServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
// 绑定端口,开始接收进来的连接
ChannelFuture f = b.bind(port).sync();
// 等待服务器 socket 关闭 。
// 在这个例子中,这不会发生,但你可以优雅地关闭你的服务器。
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
// Client端
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new ClientHandler());
}
});
// 连接服务端
ChannelFuture f = b.connect(host, port).sync();
// 等待连接关闭
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
```
2. 编写ServerHandler和ClientHandler
```java
// ServerHandler
public class ServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 处理客户端发送过来的消息
ByteBuf in = (ByteBuf) msg;
try {
System.out.println("Server received: " + in.toString(CharsetUtil.UTF_8));
// 将消息返回给客户端
ctx.write(in);
ctx.flush();
} finally {
ReferenceCountUtil.release(msg);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
// 当出现异常时关闭连接
cause.printStackTrace();
ctx.close();
}
}
// ClientHandler
public class ClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) {
// 发送消息给服务端
ctx.writeAndFlush(Unpooled.copiedBuffer("Hello Server", CharsetUtil.UTF_8));
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 处理服务端返回的消息
ByteBuf in = (ByteBuf) msg;
try {
System.out.println("Client received: " + in.toString(CharsetUtil.UTF_8));
} finally {
ReferenceCountUtil.release(msg);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
// 当出现异常时关闭连接
cause.printStackTrace();
ctx.close();
}
}
```
这样,我们就可以用Netty实现简单的即时通讯了。当然,实际应用中还需要考虑更多的因素,例如协议的定义和解析、消息的组装和拆解等。
相关推荐
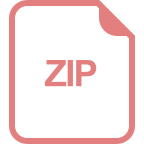
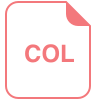
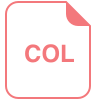
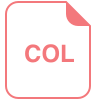
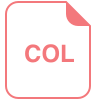
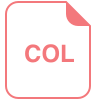







