Design a Car class contains a stop data of bool type, which indicates whether the car moves or stops.The class has thefollowing operations: 1.isMovement:move a car. When the stop data is true, a "the car moves" string is displayed; otherwise "the car stops" isdisplayed. 2.Reset: reset the stop data by overloading operator ().Requirement: 1.The class must have a copy constructor. 2.Write a test program that declares two Car objects. One of two objects is declared by one existing object and test theoperations.
时间: 2024-03-15 15:44:40 浏览: 65
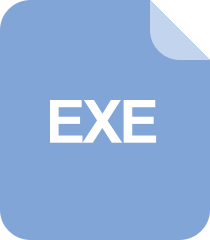
Python 查看主机IP及mac地址
Sure, I understand your question. Here is the implementation of the Car class in C++:
```cpp
#include <iostream>
class Car {
public:
Car() : stop(true) {}
Car(const Car& car) {
stop = car.stop;
}
void operator() (bool value) {
stop = value;
}
void isMovement() {
if (stop) {
std::cout << "The car moves" << std::endl;
} else {
std::cout << "The car stops" << std::endl;
}
}
private:
bool stop;
};
int main() {
Car car1;
Car car2 = car1;
car1.isMovement();
car2.isMovement();
car1(false);
car1.isMovement();
car2.isMovement();
car2(true);
car1.isMovement();
car2.isMovement();
return 0;
}
```
This program creates two Car objects: car1 and car2. The copy constructor is used to create car2 from car1. The program then tests the isMovement method by calling it on both car1 and car2, which should display "The car stops" since the stop data is initialized to true. Then, the stop data is modified using the overloaded operator (), and isMovement is called again to test the change. Finally, the stop data of car2 is modified and isMovement is called again to show that the objects are independent.
阅读全文
相关推荐
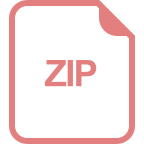
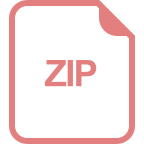
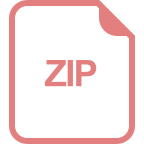
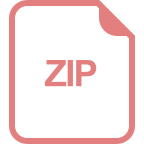
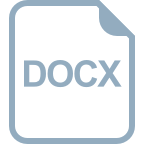
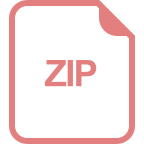
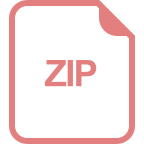
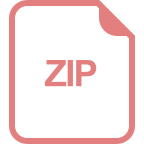
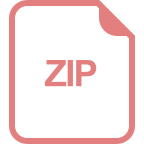
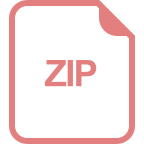