c语言、c++、Java中的字符串表示
时间: 2024-01-10 11:02:24 浏览: 95
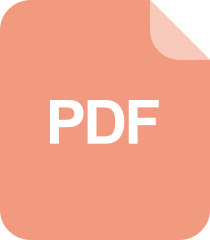
C语言中字符串的使用
在C语言中,字符串是通过字符数组来表示的,即用一组字符变量来存储一个字符串。例如:
```c
char str[] = "Hello, world!";
```
在C++中,字符串可以有多种表示方式。其中一种是使用标准库中的string类,例如:
```c++
#include <string>
using namespace std;
string str = "Hello, world!";
```
另一种是使用字符数组来表示,这和C语言中的方式是一样的。
在Java中,字符串是通过String类来表示的。例如:
```java
String str = "Hello, world!";
```
Java中的String类是一个特殊的类,它可以直接使用字符串字面量来创建对象,而不需要使用new关键字。
阅读全文
相关推荐
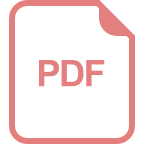
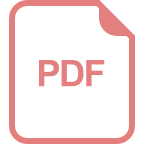
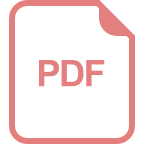
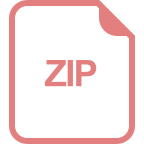
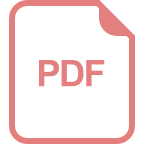
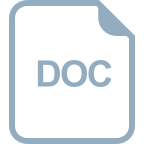
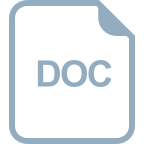
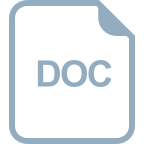
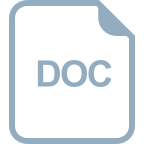
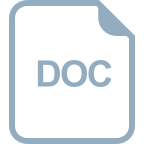
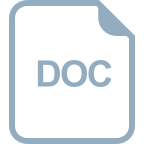

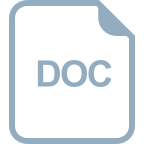
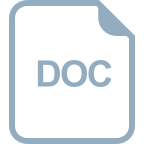
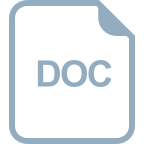
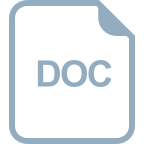
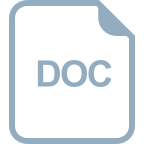
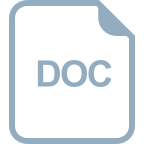