vue模糊查询,并且高亮,实现间隔搜索也可以实现
时间: 2024-01-27 22:05:50 浏览: 79
Vue 模糊查询并高亮可以通过在模板中使用 `v-html` 指令和正则表达式来实现。具体步骤如下:
1. 定义一个 computed 属性,用于过滤出匹配关键字的结果,并进行高亮处理。
```javascript
computed: {
filteredList() {
const filterKey = this.filterKey
const regExp = new RegExp(`(${filterKey})`, 'i')
return this.list.filter(item => regExp.test(item.name)).map(item => {
item.name = item.name.replace(regExp, '<span class="highlight">$1</span>')
return item
})
}
}
```
2. 在模板中使用 `v-html` 指令,将过滤后的结果渲染到页面上。
```html
<ul>
<li v-for="item in filteredList" :key="item.id" v-html="item.name"></li>
</ul>
```
3. 在 CSS 中定义高亮样式。
```css
.highlight {
color: red;
font-weight: bold;
}
```
如果你想要实现间隔搜索,可以通过在 computed 属性中设置一个计时器,在用户输入停顿一段时间后再触发搜索。具体实现可以参考下面的代码:
```javascript
computed: {
filteredList() {
const filterKey = this.filterKey
const regExp = new RegExp(`(${filterKey})`, 'i')
return this.list.filter(item => regExp.test(item.name)).map(item => {
item.name = item.name.replace(regExp, '<span class="highlight">$1</span>')
return item
})
}
},
watch: {
filterKey: function (val) {
clearTimeout(this.timer)
this.timer = setTimeout(() => {
// 等待用户输入停顿一段时间后再触发搜索
this.search()
}, 500)
}
},
methods: {
search() {
// do something
}
}
```
在模板中,你只需要绑定 `filterKey` 属性即可:
```html
<input type="text" v-model="filterKey">
<ul>
<li v-for="item in filteredList" :key="item.id" v-html="item.name"></li>
</ul>
```
阅读全文
相关推荐
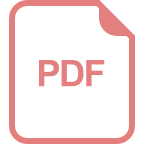
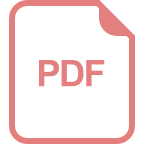
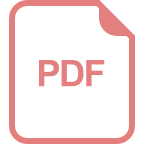
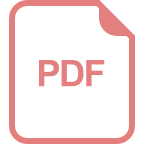
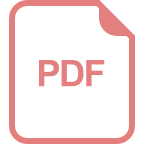
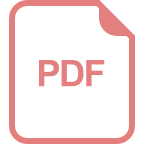
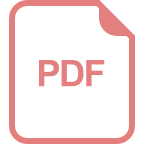
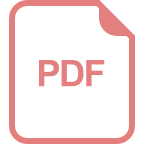
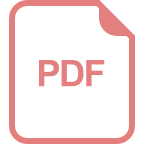
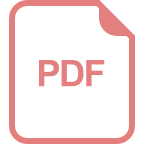
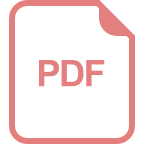
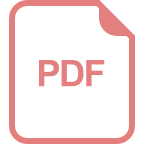
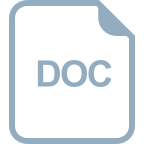
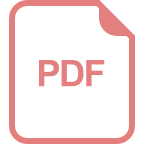
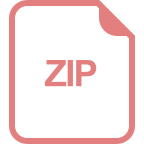
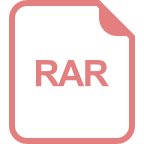
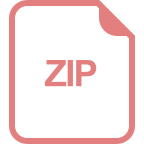