循环单链表的冒泡排序 Java语言
时间: 2023-05-27 10:05:25 浏览: 62
/**
* 循环单链表的冒泡排序
*/
public class CircularLinkedListBubbleSort {
/**
* 节点类
*/
static class Node {
int data;
Node next;
Node(int data) {
this.data = data;
}
}
/**
* 冒泡排序
* @param head 循环单链表的头节点
*/
public static void bubbleSort(Node head) {
if (head == null || head.next == null) {
return;
}
Node tail = null; // 排序区的尾节点
Node cur = head; // 当前节点
while (cur != tail) {
Node prev = null; // 当前节点的前一个节点
Node next = cur.next; // 当前节点的下一个节点
while (next != tail) {
// 如果当前节点的值大于下一个节点的值,则交换两个节点的值
if (cur.data > next.data) {
if (prev != null) {
prev.next = next;
} else {
head = next;
}
cur.next = next.next;
next.next = cur;
Node temp = next;
next = cur;
cur = temp;
}
prev = next;
next = next.next;
}
tail = cur;
cur = head;
}
}
/**
* 打印循环单链表
* @param head 循环单链表的头节点
*/
public static void printLinkedList(Node head) {
if (head == null) {
return;
}
System.out.print(head.data + " ");
Node cur = head.next;
while (cur != head) {
System.out.print(cur.data + " ");
cur = cur.next;
}
System.out.println();
}
public static void main(String[] args) {
Node head = new Node(5);
Node node1 = new Node(3);
Node node2 = new Node(9);
Node node3 = new Node(1);
Node node4 = new Node(7);
head.next = node1;
node1.next = node2;
node2.next = node3;
node3.next = node4;
node4.next = head;
System.out.println("排序前:");
printLinkedList(head);
bubbleSort(head);
System.out.println("排序后:");
printLinkedList(head);
}
}
相关推荐
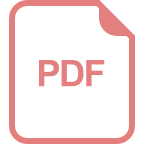
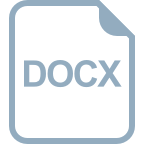
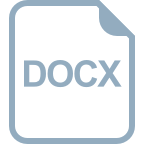














