springboot定时任务
时间: 2023-09-21 09:02:41 浏览: 40
在Spring Boot中使用定时任务非常容易,只需要使用@EnableScheduling注解开启定时任务功能,然后在需要执行定时任务的方法上加上@Scheduled注解即可。
下面是一个简单的示例:
1. 在启动类上添加@EnableScheduling注解
```java
@SpringBootApplication
@EnableScheduling
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
2. 创建一个定时任务
```java
@Component
public class MyTask {
//每隔5秒执行一次
@Scheduled(fixedRate = 5000)
public void task() {
System.out.println("定时任务执行...");
}
}
```
在上面的代码中,我们创建了一个名为MyTask的定时任务,使用@Scheduled注解指定了执行的时间间隔为5秒。
3. 运行应用程序
在完成上述步骤后,启动应用程序,就可以看到定时任务每隔5秒会执行一次。
需要注意的是,@Scheduled注解有很多属性可以用来指定任务执行的时间间隔、执行时间等,具体可以参考官方文档。
相关问题
Springboot定时任务
Spring Boot提供了多种方式来实现定时任务。以下是其中几种常用的方式:
1. 使用@Scheduled注解:在需要执行定时任务的方法上添加@Scheduled注解,并指定任务的执行时间表达式,例如:
```java
@Component
public class MyTask {
@Scheduled(cron = "0 0 0 * * ?") // 每天凌晨执行
public void execute() {
// 执行定时任务的逻辑
}
}
```
这样,Spring Boot会自动创建一个定时任务,并按照指定的时间表达式执行任务。
2. 实现SchedulingConfigurer接口:创建一个配置类,实现SchedulingConfigurer接口,重写configureTasks方法,在该方法中自定义定时任务的执行规则,例如:
```java
@Configuration
@EnableScheduling
public class MyTaskConfig implements SchedulingConfigurer {
@Override
public void configureTasks(ScheduledTaskRegistrar taskRegistrar) {
taskRegistrar.addTriggerTask(
() -> {
// 执行定时任务的逻辑
},
triggerContext -> {
CronTrigger cronTrigger = new CronTrigger("0 0 0 * * ?");
return cronTrigger.nextExecutionTime(triggerContext);
}
);
}
}
```
这样,定时任务的执行规则就由configureTasks方法中的逻辑来决定。
3. 使用Quartz框架:Spring Boot集成了Quartz框架,可以使用Quartz提供的更强大的定时任务功能。需要引入相关依赖,并配置Job和Trigger来实现定时任务的调度。
这些只是几种常用的实现方式,根据具体的需求,你可以选择适合的方式来实现Spring Boot的定时任务。
springBoot定时任务
Spring Boot提供了一种简单而强大的方式来创建和管理定时任务。你可以使用`@Scheduled`注解来标记一个方法,并指定它应该在何时执行。
首先,确保你的Spring Boot应用程序中已经配置了@EnableScheduling注解,以启用定时任务的功能。然后,在你希望定时执行的方法上添加@Scheduled注解,并指定执行的时间表达式。
时间表达式是一个字符串,用于定义定时任务的执行时间。它可以是一个固定的时间点、一个周期性的间隔,或者是一些复杂的规则。以下是一些常用的时间表达式示例:
- 固定延迟执行:@Scheduled(fixedDelay = 5000) 表示任务完成后延迟5秒再次执行。
- 固定速率执行:@Scheduled(fixedRate = 5000) 表示每隔5秒执行一次任务,不管任务是否完成。
- cron表达式:@Scheduled(cron = "0 0 12 * * ?") 表示在每天中午12点执行任务。
使用这些注解,你可以根据自己的需求创建不同类型的定时任务。
另外,还可以使用ThreadPoolTaskScheduler来配置线程池,以便在多个任务同时触发时进行并发处理。
希望这个简单的介绍能帮助到你!如有更多问题,请继续提问。
相关推荐



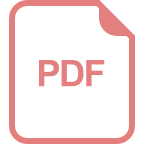
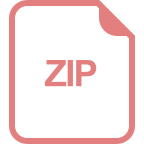
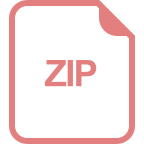
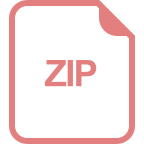
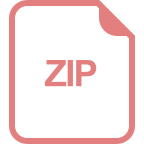
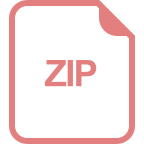