使用c#语言编写一个控制台程序,随机生成一个英文单词。游戏一开始,目标单词以星号显示,每次用户猜测其中一个字母。如果猜对了,则下一次猜时显示出该字母在目标单词中的(所有)位置。重复猜测,直到用户猜对为止。最终统计出用户猜测的次数和一次都没猜对字母的次数
时间: 2024-11-12 17:32:39 浏览: 31
在C#中编写一个简单的控制台程序,用于随机生成英文单词并进行字母猜测游戏,可以按照以下步骤操作:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
namespace WordGuessGame
{
class Program
{
static void Main(string[] args)
{
string secretWord = GetRandomWord();
char[] guessedChars = new char[secretWord.Length];
int correctGuesses = 0;
int incorrectGuesses = 0;
Console.WriteLine("Welcome to the Word Guess Game! Type 'quit' to exit.");
while (true)
{
Console.Write($"Current word is _{string.Join("_", guessedChars)}_ ");
string input = Console.ReadLine().ToUpper();
if (input == "QUIT")
break;
if (!char.IsLetter(input))
{
Console.WriteLine("Invalid input. Please enter a single letter.");
continue;
}
if (guessedChars.Any(charChar => charChar == input))
{
Console.WriteLine("You have already guessed that letter. Try again.");
continue;
}
guessedChars = ReplaceInArray(guessedChars, input);
if (IsCorrect(secretWord, guessedChars))
{
Console.WriteLine($"Congratulations! You found all the letters in {secretWord}.\n");
correctGuesses++;
PrintStats(correctGuesses, incorrectGuesses);
break;
}
else
{
Console.WriteLine("Incorrect guess. Here's the updated word:");
PrintUpdatedWord(secretWord, guessedChars);
incorrectGuesses++;
}
}
}
// Generate a random word from a predefined list or fetch it from an API
private static string GetRandomWord()
{
// Add your word list here or fetch from an external source like Wordnik API
List<string> words = new List<string>{"apple", "banana", "cherry", "orange", "kiwi"};
return words[new Random().Next(words.Count)];
}
// Replace the input character with '*' in the guessedChars array if not already present
private static char[] ReplaceInArray(char[] arr, char input)
{
for (int i = 0; i < arr.Length; i++)
{
if (arr[i] == '_')
arr[i] = input;
}
return arr;
}
// Check if the user has correctly guessed the secret word by comparing arrays
private static bool IsCorrect(string secretWord, char[] guessedChars)
{
return secretWord.SequenceEqual(guessedChars.Replace('_', string.Empty));
}
// Print statistics after the game ends
private static void PrintStats(int correctGuesses, int incorrectGuesses)
{
Console.WriteLine($"Total guesses made: {correctGuesses + incorrectGuesses}");
Console.WriteLine($"Letters not guessed at all: {secretWord.Distinct() - new string(guessedChars).Distinct()}");
}
// Print the secret word with underscores showing where each letter was guessed
private static void PrintUpdatedWord(string secretWord, char[] guessedChars)
{
Console.Write(secretWord);
}
}
}
```
在这个程序中,首先定义了一个`GetRandomWord`函数来获取一个随机单词,然后通过循环让用户输入字母进行猜测。玩家每猜对一个字母,就更新`guessedChars`数组,并检查是否全部猜对。如果猜错,会打印当前状态并继续游戏。游戏结束后,会计算正确的猜测数和未猜到的字母数。
阅读全文
相关推荐
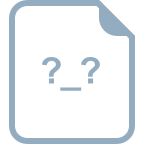
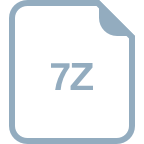
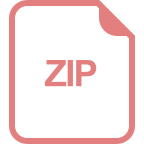
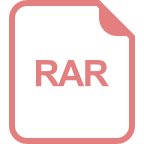
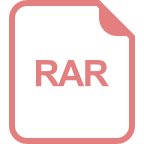
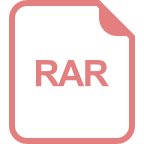
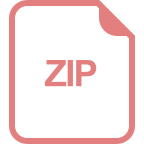
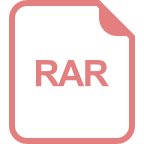
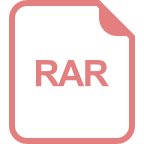
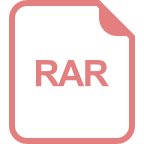
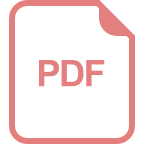
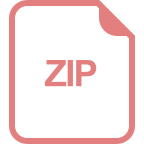
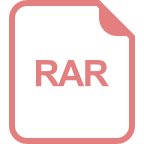
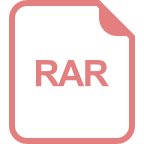
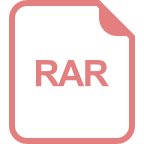
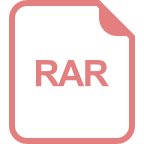
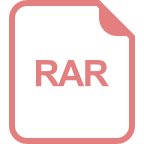
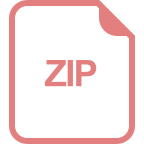
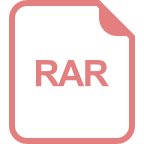