写一段用python语言和opencv库提取图像轮廓的代码
时间: 2023-03-12 21:42:04 浏览: 86
答:import cv2
img = cv2.imread('图像路径')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 50, 150)
contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(img, contours, -1, (0,255,0), 3)
cv2.imshow('result', img)
cv2.waitKey(0)
相关问题
写一段Python代码提取图像轮廓
下面是一段使用 OpenCV 库提取图像轮廓的 Python 代码:
```
import cv2
import numpy as np
# 读取图像
img = cv2.imread("image.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 使用 Canny 边缘检测算法检测边缘
edges = cv2.Canny(gray, 50, 150)
# 查找图像中的轮廓
contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 在原图上绘制轮廓
cv2.drawContours(img, contours, -1, (0, 255, 0), 2)
# 显示结果图像
cv2.imshow("Contours", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在代码中,首先我们读取了图像,并将其转换为灰度图。然后,使用 Canny 边缘检测算法检测图像中的边缘。最后,使用 `cv2.findContours()` 函数查找图像中的轮廓,并在原图上绘制出轮廓,最后显示结果图像。
用python写一段提取图像主体部分的代码
好的,以下是Python代码示例来提取图像主体部分:
```python
import cv2
# 读取图片
img = cv2.imread('your_image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 进行二值化
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
# 获取边缘
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnt = max(contours, key=cv2.contourArea)
# 获取矩形框坐标
x, y, w, h = cv2.boundingRect(cnt)
# 裁剪图像
crop = img[y:y + h, x:x + w]
# 显示结果
cv2.imshow('crop', crop)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码使用 OpenCV 库来提取图像主体部分。它将图像转换为灰度图像,进行二值化,并查找图像中的轮廓。接着,它找到面积最大的轮廓,并从中提取矩形框坐标。最后,它对原始图像进行裁剪,只保留主体部分,并将结果显示出来。
相关推荐
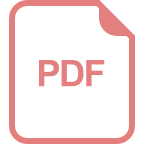
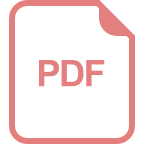
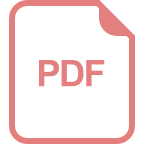
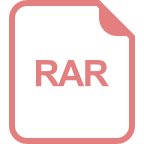
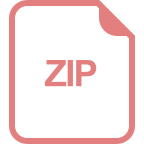
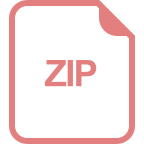
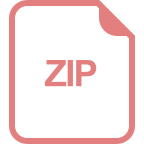
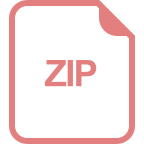
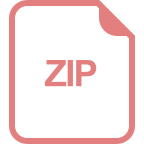