字符串转byte数组
时间: 2023-06-28 17:11:24 浏览: 44
在Java中,可以使用字符串的 `getBytes()` 方法将字符串转换为字节数组。例如:
```java
String str = "hello";
byte[] bytes = str.getBytes();
```
上述代码将字符串 `"hello"` 转换为字节数组 `bytes`。如果需要指定字符集,则可以在 `getBytes()` 方法中传递字符集参数。例如:
```java
String str = "你好";
byte[] bytes = str.getBytes("UTF-8");
```
上述代码将字符串 `"你好"` 使用 UTF-8 编码转换为字节数组 `bytes`。
相关问题
字符串转 byte数组
在Java中,有几种方法可以将字符串转换为字节数组。
一种常见的方法是使用String类的getBytes()方法。这个方法有多个重载形式,其中一个常用的形式是getBytes(String charsetName),它可以将字符串按照指定的字符集编码为字节数组。例如,可以使用UTF-8字符集将字符串转换为字节数组,代码如下:
String str = "Hello World";
byte[] byteArray = str.getBytes("UTF-8");
还有另一种方法是使用Charset类的encode()方法。这个方法也可以将字符串按照指定的字符集编码为字节数组。例如,可以使用UTF-8字符集将字符串转换为字节数组,代码如下:
String str = "Hello World";
Charset charset = Charset.forName("UTF-8");
ByteBuffer byteBuffer = charset.encode(str);
byte[] byteArray = byteBuffer.array();
这些方法可以根据实际情况选择合适的转换方法和字符集。
字符串 转byte数组
要将字符串转换为字节数组,你可以使用字符串的 `getBytes()` 方法。这个方法将返回一个字节数组,其中包含了字符串的字节表示。
以下是一个示例代码:
```java
String str = "Hello, World!";
byte[] byteArray = str.getBytes();
```
在上面的代码中,我们将字符串 "Hello, World!" 转换为字节数组 `byteArray`。现在,你可以使用这个字节数组进行进一步的处理或传输。
相关推荐
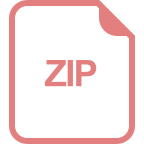
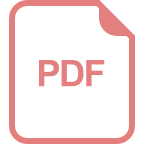












