编写C语言代码,实现高响应比优先调度算法
时间: 2024-10-25 13:05:06 浏览: 158
在C语言中,实现高响应比优先(HRRN,Highest Response Ratio Next)调度算法需要创建一个任务结构体,以及用于维护任务状态的数据结构。以下是简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 定义任务结构体
typedef struct Task {
int id;
float burst_time; // 执行时间
float response_time; // 当前已运行时间
} Task;
// 比较函数,用于计算响应比
int compare(const void *a, const void *b) {
Task *taskA = (Task *)a;
Task *taskB = (Task *)b;
float ratioA = taskA->response_time / taskA->burst_time;
float ratioB = taskB->response_time / taskB->burst_time;
return ratioB - ratioA; // 高响应比的任务排在前面
}
// HRRN调度函数
void hrrn_scheduling(Task tasks[], int n) {
qsort(tasks, n, sizeof(Task), compare); // 排序任务
for (int i = 0; i < n; i++) {
printf("Task %d: Burst Time = %f, Current Time = %f\n", tasks[i].id, tasks[i].burst_time, tasks[i].response_time);
// 假设每个任务都按顺序执行
sleep(tasks[i].burst_time);
tasks[i].response_time += tasks[i].burst_time; // 更新响应时间
}
}
int main() {
int num_tasks;
printf("Enter the number of tasks: ");
scanf("%d", &num_tasks);
Task tasks[num_tasks];
for (int i = 0; i < num_tasks; i++) {
printf("Enter details for Task %d: ID and Burst Time\n", i + 1);
scanf("%d%f", &tasks[i].id, &tasks[i].burst_time);
tasks[i].response_time = 0; // 初始响应时间为0
}
hrrn_scheduling(tasks, num_tasks);
return 0;
}
```
阅读全文
相关推荐
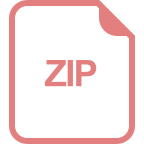
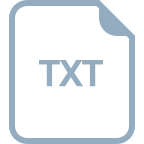
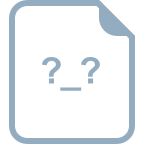



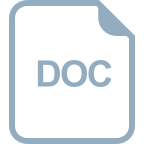









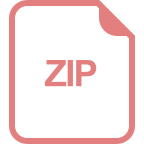