all the input array dimensions for the concatenation axis must match exactly, but along dimension 0, the array at index 0 has size 14 and the array at index 1 has size 249
时间: 2024-02-27 19:56:26 浏览: 483
这个错误提示是由于使用`numpy.concatenate`函数进行数组拼接时,拼接的数组在拼接的轴上的维度不匹配导致的。具体来说,在axis=0的轴上,数组的大小不相同,第一个数组大小为14,而第二个数组大小为249。
为了解决这个问题,我们需要保证拼接的数组的大小在拼接轴上具有相同的维度。可以通过对其中一个数组进行切片操作,或者使用`numpy.reshape`函数来改变数组的大小,使其能够与另一个数组在拼接轴上具有相同的维度。
例如,如果我们想要将大小为14的数组`a`和大小为249的数组`b`在axis=0的轴上进行拼接,可以使用以下代码:
```
import numpy as np
# 创建大小为14的数组a
a = np.random.rand(14, 2)
# 创建大小为249的数组b
b = np.random.rand(249, 2)
# 将大小为14的数组a在axis=0的轴上进行重复,使其大小与数组b的大小相同
a_repeated = np.repeat(a, 18, axis=0)
# 将两个数组在axis=0的轴上进行拼接
result = np.concatenate((a_repeated, b), axis=0)
```
在上述代码中,我们使用了`numpy.repeat`函数将大小为14的数组`a`在axis=0的轴上进行了重复,使其大小与数组`b`的大小相同,从而使得两个数组在axis=0的轴上具有相同的大小。最终结果存储在`result`变量中。
相关问题
all the input array dimensions except for the concatenation axis must match exactly, but along dimension 0, the array at index 0 has size 3 and the array at index 1 has size 2
这个错误是由于在使用`np.hstack`函数进行连接时,要求除了连接轴之外的维度必须完全匹配。根据错误信息,你的输入数据在第0维上的大小不匹配。
例如,在以下情况下会引发这个错误:
```python
import numpy as np
input_data_1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
output_data_1 = np.array([[10, 11], [12, 13], [14, 15]])
input_data_2 = np.array([[2, 3], [5, 6], [8, 9]])
output_data_2 = np.array([[11, 12], [13, 14], [15, 16]])
A_eq = np.hstack((input_data_1.T, -output_data_1.T, input_data_2.T, -output_data_2.T))
```
在这个例子中,`input_data_1`的大小是3x3,`output_data_1`的大小是3x2,而`input_data_2`的大小是3x2,`output_data_2`的大小也是3x2。由于`output_data_1`和`output_data_2`在第0维上的大小不匹配,所以会引发上述错误。
要解决这个问题,你需要确保输入数据在所有维度上的大小匹配。如果你有任何其他问题或更多的信息,请随时告诉我。
all the input array dimensions except for the concatenation axis must match exactly,but along dimension 0,the array at index 0 has size 2922 and the array at index 1 has size 24这个错误要怎么改
这个错误通常是在使用numpy的concatenate函数时出现的,因为concatenate函数要求除了连接轴之外的其他维度必须完全匹配。在这个错误中,数组在第0维的大小不匹配。
要解决这个错误,你需要检查数组的形状,并确保它们在连接轴之外的所有维度都完全匹配。如果你想在第0维上连接这些数组,你需要使用reshape函数来改变数组的大小,以使它们在第0维上具有相同的大小。
如果你想在第0维上连接这些数组,你可以使用以下代码:
```
import numpy as np
# 假设a和b是你要连接的两个数组
a = np.random.rand(2922, 3)
b = np.random.rand(24, 3)
# 将b数组的大小改变为(1, 24, 3)
b = np.reshape(b, (1, 24, 3))
# 在第0维上连接a和b
c = np.concatenate((a, b), axis=0)
```
注意,在将b数组的大小改变为(1, 24, 3)之后,它就可以与大小为(2922, 3)的a数组在第0维上连接了。
阅读全文
相关推荐


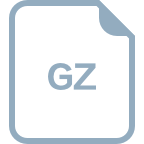






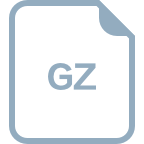

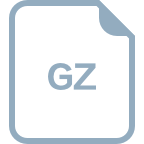
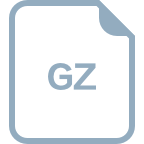