设计Java程序完成: 1.文件类。(1).完成拷贝任意类型的文件。 (2).完成目录拷贝,包括子目录及各个文件。 2.消息类。(1)包括发送人,接受人,发消息的时间、消息内容等。 (2)把若干个消息对象存到文件中。 (3)从文件中读入若干消息对象,并输出到控制台。
时间: 2024-10-01 17:02:41 浏览: 15
设计Java程序来完成这两个任务可以分为两部分:
1. 文件类:
```java
import java.io.*;
public class FileHandler {
// 完成拷贝任意类型的文件
public static void copyFile(Object source, String srcPath, Object dest, String dstPath) throws IOException {
if (source instanceof FileInputStream && dest instanceof FileOutputStream) {
FileInputStream fis = (FileInputStream) source;
FileOutputStream fos = (FileOutputStream) dest;
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) != -1) {
fos.write(buffer, 0, length);
}
fis.close();
fos.close();
} else {
throw new IllegalArgumentException("Unsupported file types");
}
}
// 完成目录拷贝
public static void copyDirectory(String srcDir, String dstDir) throws IOException {
File src = new File(srcDir);
if (!src.exists() || !src.isDirectory()) {
throw new FileNotFoundException("Source directory not found or invalid");
}
File dst = new File(dstDir);
if (!dst.exists()) {
dst.mkdirs();
}
for (File file : src.listFiles()) {
if (file.isDirectory()) {
copyDirectory(file.getAbsolutePath(), dstDir + "/" + file.getName());
} else {
copyFile(file, file.getAbsolutePath(), new File(dst, file.getName()), file.getAbsolutePath());
}
}
}
}
```
2. 消息类:
```java
import java.time.LocalDateTime;
public class Message {
private String sender;
private String receiver;
private LocalDateTime timestamp;
private String content;
// 构造函数、getters和setters省略...
@Override
public String toString() {
return "Message{" +
"sender='" + sender + '\'' +
", receiver='" + receiver + '\'' +
", timestamp=" + timestamp +
", content='" + content + '\'' +
'}';
}
}
// 存储和读取消息
import java.util.ArrayList;
import java.util.List;
public class MessageManager {
public List<Message> saveMessagesToFile(List<Message> messages, String filePath) throws IOException {
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(filePath))) {
oos.writeObject(messages);
}
return messages;
}
public static List<Message> loadMessagesFromFile(String filePath) throws IOException {
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filePath))) {
return (List<Message>) ois.readObject();
}
}
}
```
阅读全文
相关推荐
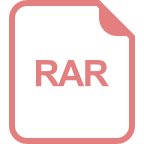
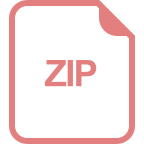
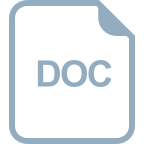















